单元最短路径算法C++
时间: 2023-11-08 20:06:19 浏览: 97
单元最短路径算法有多种实现方式,其中比较常用的是Dijkstra算法和Bellman-Ford算法。下面给出它们的C++实现。
1. Dijkstra算法
Dijkstra算法用于求解从起点到终点的最短路径,要求边的权值非负。算法的基本思想是从起点开始,每次找到当前距离起点最近的一个点,然后用该点更新其它点的距离,直到到达终点或者所有点都被访问过。
下面是Dijkstra算法的C++实现:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <cstring>
#include <algorithm>
using namespace std;
const int N = 100010, INF = 0x3f3f3f3f;
int n, m, s, t;
int h[N], e[N], w[N], ne[N], idx;
int dist[N];
bool st[N];
void add(int a, int b, int c)
{
e[idx] = b, w[idx] = c, ne[idx] = h[a], h[a] = idx ++ ;
}
void dijkstra()
{
memset(dist, 0x3f, sizeof dist);
dist[s] = 0;
priority_queue<pair<int, int>, vector<pair<int, int>>, greater<>> q;
q.push({0, s});
while (q.size())
{
auto t = q.top();
q.pop();
int ver = t.second, distance = t.first;
if (st[ver]) continue;
st[ver] = true;
for (int i = h[ver]; ~i; i = ne[i])
{
int j = e[i];
if (dist[j] > distance + w[i])
{
dist[j] = distance + w[i];
q.push({dist[j], j});
}
}
}
}
int main()
{
memset(h, -1, sizeof h);
cin >> n >> m >> s >> t;
while (m -- )
{
int a, b, c;
cin >> a >> b >> c;
add(a, b, c);
}
dijkstra();
cout << (dist[t] == INF ? -1 : dist[t]) << endl;
return 0;
}
```
2. Bellman-Ford算法
Bellman-Ford算法用于求解任意两点之间的最短路径,要求边的权值可以是负数。算法的基本思想是对所有的边进行n-1次松弛操作,其中n是图中点的个数。如果在第n-1次松弛操作后仍然存在松弛的情况,说明图中存在负环,即从起点出发可以无限循环地减小距离。
下面是Bellman-Ford算法的C++实现:
```c++
#include <iostream>
#include <vector>
#include <cstring>
#include <algorithm>
using namespace std;
const int N = 100010, INF = 0x3f3f3f3f;
int n, m, s, t;
int h[N], e[N], w[N], ne[N], idx;
int dist[N], cnt[N];
bool st[N];
void add(int a, int b, int c)
{
e[idx] = b, w[idx] = c, ne[idx] = h[a], h[a] = idx ++ ;
}
bool spfa()
{
memset(dist, 0x3f, sizeof dist);
dist[s] = 0;
queue<int> q;
q.push(s);
st[s] = true;
cnt[s] = 1;
while (q.size())
{
int t = q.front();
q.pop();
st[t] = false;
for (int i = h[t]; ~i; i = ne[i])
{
int j = e[i];
if (dist[j] > dist[t] + w[i])
{
dist[j] = dist[t] + w[i];
cnt[j] = cnt[t] + 1;
if (cnt[j] >= n) return true;
if (!st[j])
{
q.push(j);
st[j] = true;
}
}
}
}
return false;
}
int main()
{
memset(h, -1, sizeof h);
cin >> n >> m >> s >> t;
while (m -- )
{
int a, b, c;
cin >> a >> b >> c;
add(a, b, c);
}
if (spfa()) puts("-1");
else cout << dist[t] << endl;
return 0;
}
```
阅读全文
相关推荐
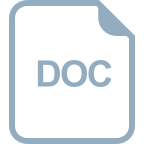
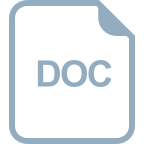
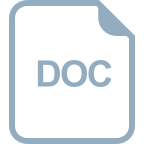

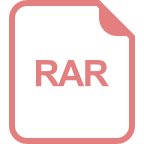
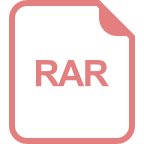
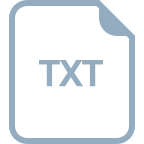
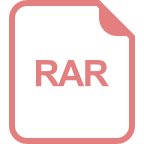




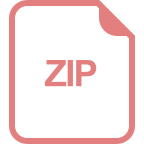
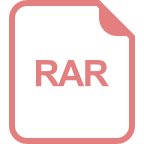
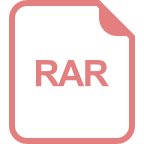
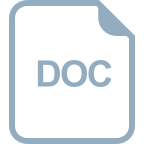
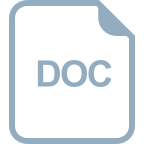
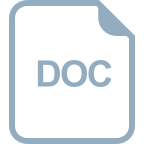