C# 设置软件管理员权限
时间: 2023-07-27 08:26:53 浏览: 58
在 C# 中,你可以通过以下代码设置软件的管理员权限:
```csharp
using System.Diagnostics;
using System.Security.Principal;
// 检查当前用户是否为管理员
bool isAdmin = new WindowsPrincipal(WindowsIdentity.GetCurrent()).IsInRole(WindowsBuiltInRole.Administrator);
if (!isAdmin)
{
// 创建一个启动信息对象
ProcessStartInfo startInfo = new ProcessStartInfo();
// 指定要启动的程序的路径和文件名
startInfo.FileName = Application.ExecutablePath;
// 指定启动时要使用的用户名和密码
startInfo.UserName = "Administrator";
startInfo.Password = new SecureString();
string password = "123456"; // 这里需要替换为实际的管理员密码
foreach (char c in password)
{
startInfo.Password.AppendChar(c);
}
// 指定启动时使用的域名,默认为本地机器名
startInfo.Domain = Environment.MachineName;
// 指定启动时的参数
startInfo.Arguments = "/restart";
// 指定启动时的工作目录
startInfo.WorkingDirectory = Application.StartupPath;
// 指定启动时的窗口模式
startInfo.WindowStyle = ProcessWindowStyle.Normal;
// 指定启动时的标准输入输出流
startInfo.UseShellExecute = false;
startInfo.RedirectStandardInput = true;
startInfo.RedirectStandardOutput = true;
// 启动程序,并等待程序退出
Process process = new Process();
process.StartInfo = startInfo;
process.Start();
process.WaitForExit();
}
```
这段代码首先检查当前用户是否为管理员,如果不是,则使用 `ProcessStartInfo` 对象来启动程序,并使用管理员权限运行程序。在 `ProcessStartInfo` 对象中,我们可以指定启动时使用的用户名、密码、域名、参数、工作目录、窗口模式以及标准输入输出流等信息。最后,我们使用 `Process` 对象启动程序,并等待程序退出。
需要注意的是,使用管理员权限运行程序需要输入管理员账户的密码,这里我们将密码以明文的方式写在代码中,这并不是一个安全的做法。为了保证安全性,我们应该将密码以加密的方式保存在配置文件中,或者使用其他安全的方式来获取管理员密码。
相关推荐
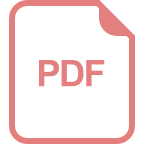
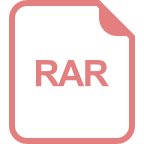
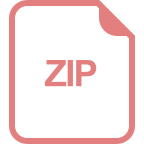














