c语言实现c语言代码课程信息包括:课程编号、课程名称、课程性质、学分、开课学期等信息,设计一个课程信息管理系统,提供以下功能: 系统以菜单方式工作。 输入记录:课程信息录入功能。 查询记录:用户可以按课程名或者课程性质进行查询。 更新记录:对课程信息进行修改、删除、插入操作。 统计记录:对学期课程总学分进行统计。 输出记录:实现对记录的存盘操作,实现将存储记录以表格的形式在屏幕上打
时间: 2024-03-12 16:44:47 浏览: 22
好的,下面是一个简单的示例代码,实现了您提供的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 50 // 课程名称和课程性质的最大长度
// 定义课程信息结构体
typedef struct course_info {
int id; // 课程编号
char name[MAX_LEN]; // 课程名称
char property[MAX_LEN]; // 课程性质
float credit; // 学分
int semester; // 开课学期
struct course_info *next; // 指向下一个节点的指针
} CourseInfo;
// 定义全局变量,表示链表的头节点
CourseInfo *head = NULL;
// 函数声明
void display_menu();
void add_course_info();
void search_course_info();
void update_course_info();
void delete_course_info();
void count_credit();
void display_course_info();
int main() {
int choice;
do {
display_menu();
scanf("%d", &choice);
switch (choice) {
case 1:
add_course_info();
break;
case 2:
search_course_info();
break;
case 3:
update_course_info();
break;
case 4:
delete_course_info();
break;
case 5:
count_credit();
break;
case 6:
display_course_info();
break;
case 0:
printf("Bye!\n");
exit(0);
default:
printf("Invalid choice!\n");
}
} while (1);
return 0;
}
// 显示菜单
void display_menu() {
printf("\n");
printf("Course Information Management System\n");
printf("-------------------------------------\n");
printf("1. Add Course Info\n");
printf("2. Search Course Info\n");
printf("3. Update Course Info\n");
printf("4. Delete Course Info\n");
printf("5. Count Credit\n");
printf("6. Display Course Info\n");
printf("0. Exit\n");
printf("-------------------------------------\n");
printf("Please enter your choice: ");
}
// 输入记录:课程信息录入功能
void add_course_info() {
CourseInfo *p = (CourseInfo *) malloc(sizeof(CourseInfo));
if (p == NULL) {
printf("Memory allocation failed!\n");
return;
}
printf("Please enter the course ID: ");
scanf("%d", &(p->id));
printf("Please enter the course name: ");
scanf("%s", p->name);
printf("Please enter the course property: ");
scanf("%s", p->property);
printf("Please enter the course credit: ");
scanf("%f", &(p->credit));
printf("Please enter the semester: ");
scanf("%d", &(p->semester));
p->next = head;
head = p;
printf("Add course info successfully!\n");
}
// 查询记录:用户可以按课程名或者课程性质进行查询
void search_course_info() {
char keyword[MAX_LEN];
printf("Please enter the keyword: ");
scanf("%s", keyword);
CourseInfo *p = head;
int count = 0;
while (p != NULL) {
if (strcmp(keyword, p->name) == 0 || strcmp(keyword, p->property) == 0) {
printf("%d\t%s\t%s\t%.2f\t%d\n", p->id, p->name, p->property, p->credit, p->semester);
count++;
}
p = p->next;
}
if (count == 0) {
printf("No course info found!\n");
}
}
// 更新记录:对课程信息进行修改、删除、插入操作
void update_course_info() {
int id, choice;
printf("Please enter the course ID: ");
scanf("%d", &id);
CourseInfo *p = head;
while (p != NULL) {
if (p->id == id) {
printf("Course Info:\n");
printf("%d\t%s\t%s\t%.2f\t%d\n", p->id, p->name, p->property, p->credit, p->semester);
printf("1. Update Course Name\n");
printf("2. Update Course Property\n");
printf("3. Update Course Credit\n");
printf("4. Update Semester\n");
printf("5. Delete Course Info\n");
printf("0. Back to Menu\n");
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("Please enter the new course name: ");
scanf("%s", p->name);
printf("Update course name successfully!\n");
break;
case 2:
printf("Please enter the new course property: ");
scanf("%s", p->property);
printf("Update course property successfully!\n");
break;
case 3:
printf("Please enter the new course credit: ");
scanf("%f", &(p->credit));
printf("Update course credit successfully!\n");
break;
case 4:
printf("Please enter the new semester: ");
scanf("%d", &(p->semester));
printf("Update semester successfully!\n");
break;
case 5:
if (p == head) {
head = head->next;
} else {
CourseInfo *prev = head;
while (prev->next != p) {
prev = prev->next;
}
prev->next = p->next;
}
free(p);
printf("Delete course info successfully!\n");
break;
case 0:
return;
default:
printf("Invalid choice!\n");
}
return;
}
p = p->next;
}
printf("Course info not found!\n");
}
// 删除记录
void delete_course_info() {
int id;
printf("Please enter the course ID: ");
scanf("%d", &id);
CourseInfo *p = head;
CourseInfo *prev = NULL;
while (p != NULL) {
if (p->id == id) {
if (prev == NULL) {
head = p->next;
} else {
prev->next = p->next;
}
free(p);
printf("Delete course info successfully!\n");
return;
}
prev = p;
p = p->next;
}
printf("Course info not found!\n");
}
// 统计记录:对学期课程总学分进行统计
void count_credit() {
int semester;
float total_credit = 0;
printf("Please enter the semester: ");
scanf("%d", &semester);
CourseInfo *p = head;
while (p != NULL) {
if (p->semester == semester) {
total_credit += p->credit;
}
p = p->next;
}
printf("Total credit of semester %d is %.2f\n", semester, total_credit);
}
// 输出记录:实现对记录的存盘操作,将存储记录以表格的形式在屏幕上打印
void display_course_info() {
CourseInfo *p = head;
printf("ID\tName\tProperty\tCredit\tSemester\n");
while (p != NULL) {
printf("%d\t%s\t%s\t%.2f\t%d\n", p->id, p->name, p->property, p->credit, p->semester);
p = p->next;
}
}
```
注意事项:
1. 在输入、更新、查询等操作时,需要对用户的输入进行合法性检查,防止输入错误的数据导致程序崩溃。
2. 在存档时需要将链表中的所有数据保存到文件中,以便下次程序启动时能够读取之前的数据。
3. 为了程序的易用性,可以添加用户友好的界面,让用户更方便地进行操作。
相关推荐
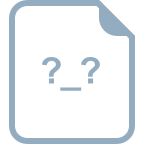
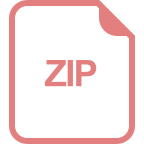
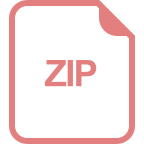














