运用C语言将两个字符串连接起来,不要用strcat函数。
时间: 2023-12-15 12:12:45 浏览: 82
可以使用指针来实现字符串连接,示例代码如下:
```c
#include <stdio.h>
void my_strcat(char *s1, char *s2) {
// 将指针移动到s1的末尾
while (*s1 != '\0') {
s1++;
}
// 逐个将s2的字符添加到s1的末尾
while (*s2 != '\0') {
*s1 = *s2;
s1++;
s2++;
}
// 添加字符串结束符
*s1 = '\0';
}
int main() {
char s1[20] = "hello";
char s2[] = "world";
my_strcat(s1, s2);
printf("%s\n", s1); // 输出 "helloworld"
return 0;
}
```
在上面的代码中,我们先将指针移动到`s1`的末尾(即`\0`字符所在的位置),然后逐个将`s2`的字符添加到`s1`的末尾,最后再添加一个字符串结束符`\0`。这样就实现了字符串的连接。
相关问题
c语言将两个字符串连接起来不要用strcat函数
可以使用循环将第二个字符串中的每个字符添加到第一个字符串的末尾,直到第二个字符串的结尾标志'\0'。例如:
```c
#include <stdio.h>
void mystrcat(char *s1, const char *s2) {
while (*s1) { // 找到s1的结尾
s1++;
}
while (*s2) { // 将s2中的字符添加到s1的末尾
*s1 = *s2;
s1++;
s2++;
}
*s1 = '\0'; // 添加字符串结尾标志
}
int main() {
char s1[20] = "Hello, ";
char s2[] = "world!";
mystrcat(s1, s2);
printf("%s\n", s1); // 输出Hello, world!
return 0;
}
```
用c语言将两个字符串连接起来,不要用strcat函数。
在C语言中,如果你想将两个字符串连接起来而不使用`strcat()`函数,你可以手动操作字符数组。首先,你需要确定目标字符串的总长度(包括最后一个空字符`\0`),然后在内存中逐个复制第二个字符串的字符到第一个字符串的末尾。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义一个函数用于连接两个字符串
char* concatenateStrings(char* str1, char* str2) {
// 获取str1的长度,不包括'\0'
int len1 = strlen(str1);
// 计算str2的长度,不包括'\0'
int len2 = strlen(str2);
// 确保有足够的空间存储两个字符串和终止符
char* result = (char*)malloc(len1 + len2 + 1); // +1 for the null terminator
// 将str1复制到result
memcpy(result, str1, len1);
// 将str2的字符追加到str1的末尾
for (int i = 0; i < len2; ++i) {
result[len1 + i] = str2[i];
}
// 结束位置添加终止符
result[len1 + len2] = '\0';
return result;
}
int main() {
char str1[] = "Hello, ";
char str2[] = "world!";
char* combined = concatenateStrings(str1, str2);
printf("Combined string: %s\n", combined);
free(combined); // 别忘了释放动态分配的内存
return 0;
}
```
在这个例子中,我们创建了一个新的字符串`combined`,并手动将`str1`和`str2`的内容连接在一起。
阅读全文
相关推荐
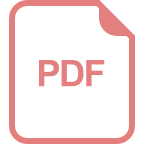
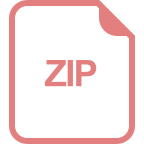
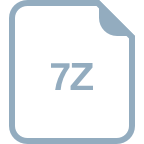













