python画散点图并找到线性回归
时间: 2023-09-06 17:13:34 浏览: 45
要绘制散点图并找到线性回归,可以使用Python中的matplotlib库和scikit-learn库。
首先,安装这两个库:
```
pip install matplotlib
pip install scikit-learn
```
然后,导入所需的库:
```python
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
```
接下来,创建一些随机数据并绘制散点图:
```python
import numpy as np
# 创建随机数据
x = np.array([1, 2, 3, 4, 5])
y = np.array([2, 4, 5, 4, 5])
# 绘制散点图
plt.scatter(x, y)
plt.show()
```
这将显示一个散点图,其中x轴是输入数据,y轴是输出数据。
接下来,使用sklearn库中的LinearRegression模型来拟合数据并绘制线性回归线:
```python
# 使用线性回归模型拟合数据
model = LinearRegression().fit(x.reshape(-1, 1), y)
# 绘制散点图和线性回归线
plt.scatter(x, y)
plt.plot(x, model.predict(x.reshape(-1, 1)), color='red')
plt.show()
```
这将显示一个带有线性回归线的散点图,该线表示x和y之间的线性关系。
相关问题
python散点图线性回归
要在Python中制作散点图和进行线性回归,你可以使用matplotlib和sklearn库。以下是一种实现方法:
首先,导入所需的库:
import numpy as np
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
然后,准备数据并绘制散点图:
x = np.array([2, 5, 8, 8, 13, 15, 17, 19, 21, 24])
y = np.array([12, 31, 45, 52, 79, 85, 115, 119, 135, 145])
plt.scatter(x, y)
plt.xlabel("battery")
plt.ylabel("KM")
plt.title("散点图")
plt.show()
接下来,建立线性回归模型并进行拟合:
regressor = LinearRegression()
regressor.fit(np.reshape(x, (-1, 1)), np.reshape(y, (-1, 1)))
打印拟合结果(参数):
print("斜率:", regressor.coef_)
print("截距:", regressor.intercept_)
最后,画出数据和拟合直线的图:
plt.scatter(x, y)
plt.plot(np.reshape(x, (-1, 1)), regressor.predict(np.reshape(x, (-1, 1))))
plt.xlabel("battery")
plt.ylabel("KM")
plt.title("拟合结果")
plt.show()
这样,你就可以在Python中绘制散点图和进行线性回归了。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [python 编程 线性回归,散点图,拟合结果](https://blog.csdn.net/qq_42262610/article/details/124290573)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [Python机器学习——线性回归案例(一)](https://blog.csdn.net/lucky_shi/article/details/105186572)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
python线性回归散点图
下面是使用Python中matplotlib库绘制线性回归散点图的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
np.random.seed(0)
x = np.random.rand(50)
y = 2*x + np.random.normal(0, 0.1, 50)
# 绘制散点图
plt.scatter(x, y, color='blue')
# 计算线性回归方程
slope, intercept = np.polyfit(x, y, 1)
x_fit = np.linspace(0, 1, 50)
y_fit = slope * x_fit + intercept
# 绘制回归直线
plt.plot(x_fit, y_fit, color='red')
# 设置图形标题和轴标签
plt.title('Linear Regression Scatter Plot')
plt.xlabel('X')
plt.ylabel('Y')
# 显示图形
plt.show()
```
解释一下代码的思路:
1. 生成随机的x和y数据。
2. 使用plt.scatter()函数绘制散点图。
3. 使用np.polyfit()函数计算线性回归方程的斜率和截距。
4. 使用np.linspace()函数生成一组x值,用于绘制回归直线。
5. 根据线性回归方程计算对应的y值。
6. 使用plt.plot()函数绘制回归直线。
7. 使用plt.title()、plt.xlabel()和plt.ylabel()函数设置图形标题和轴标签。
8. 使用plt.show()函数显示图形。
相关推荐
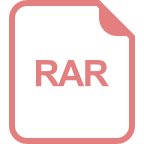
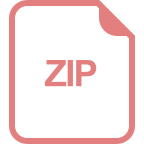
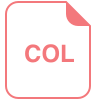
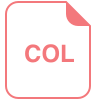
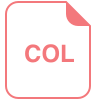
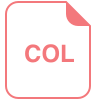
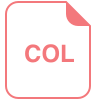







