python rsa对txt进行加密解密
时间: 2023-07-04 07:14:48 浏览: 116
要使用 Python 进行 RSA 加密和解密,我们需要使用 `pycryptodome` 库。可以通过 `pip` 安装:
```
pip install pycryptodome
```
下面是一个简单的示例,演示如何使用 RSA 进行文件加密和解密:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 生成RSA密钥对
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
# 将公钥和私钥保存到磁盘
with open('private_key.pem', 'wb') as f:
f.write(private_key)
with open('public_key.pem', 'wb') as f:
f.write(public_key)
# 从磁盘加载公钥和私钥
with open('private_key.pem', 'rb') as f:
private_key = RSA.import_key(f.read())
with open('public_key.pem', 'rb') as f:
public_key = RSA.import_key(f.read())
# 加密文件
def encrypt_file(filename, public_key):
with open(filename, 'rb') as f:
data = f.read()
cipher_rsa = PKCS1_OAEP.new(public_key)
encrypted_data = cipher_rsa.encrypt(data)
with open(filename + '.enc', 'wb') as f:
f.write(encrypted_data)
# 解密文件
def decrypt_file(filename, private_key):
with open(filename, 'rb') as f:
encrypted_data = f.read()
cipher_rsa = PKCS1_OAEP.new(private_key)
data = cipher_rsa.decrypt(encrypted_data)
with open(filename[:-4], 'wb') as f:
f.write(data)
# 加密文件
encrypt_file('plaintext.txt', public_key)
# 解密文件
decrypt_file('plaintext.txt.enc', private_key)
```
在这个示例中,我们首先生成了一个 RSA 密钥对,并将公钥和私钥保存到磁盘。然后,我们使用 `PKCS1_OAEP` 加密方案,将明文文件加密,并将加密后的数据保存到磁盘。最后,我们使用相同的私钥对加密后的文件进行解密,并将解密后的数据保存到磁盘。
阅读全文
相关推荐
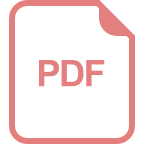

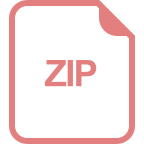
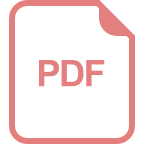
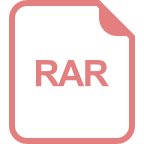






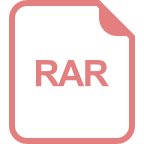
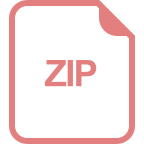
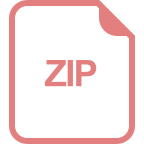