帮我用Java写一个五子棋
时间: 2023-05-17 18:03:56 浏览: 53
好的,以下是一个简单的五子棋游戏的 Java 代码:
```
import java.util.Scanner;
public class Gomoku {
private static final int BOARD_SIZE = 15;
private static final char EMPTY = '-';
private static final char BLACK = 'X';
private static final char WHITE = 'O';
private static char[][] board = new char[BOARD_SIZE][BOARD_SIZE];
private static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
initBoard();
printBoard();
char currentPlayer = BLACK;
while (true) {
System.out.println("Player " + currentPlayer + "'s turn:");
int row = getRow();
int col = getCol();
if (isValidMove(row, col)) {
board[row][col] = currentPlayer;
printBoard();
if (isWinningMove(row, col)) {
System.out.println("Player " + currentPlayer + " wins!");
break;
}
if (isBoardFull()) {
System.out.println("Game over, it's a tie!");
break;
}
currentPlayer = (currentPlayer == BLACK) ? WHITE : BLACK;
} else {
System.out.println("Invalid move, please try again.");
}
}
}
private static void initBoard() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
board[i][j] = EMPTY;
}
}
}
private static void printBoard() {
System.out.print(" ");
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < BOARD_SIZE; i++) {
System.out.print(i + " ");
for (int j = 0; j < BOARD_SIZE; j++) {
System.out.print(board[i][j] + " ");
}
System.out.println();
}
}
private static int getRow() {
System.out.print("Enter row (0-" + (BOARD_SIZE - 1) + "): ");
return scanner.nextInt();
}
private static int getCol() {
System.out.print("Enter column (0-" + (BOARD_SIZE - 1) + "): ");
return scanner.nextInt();
}
private static boolean isValidMove(int row, int col) {
return (row >= 0 && row < BOARD_SIZE && col >= 0 && col < BOARD_SIZE && board[row][col] == EMPTY);
}
private static boolean isWinningMove(int row, int col) {
char player = board[row][col];
int count = 0;
// check horizontal
for (int i = Math.max(0, col - 4); i <= Math.min(BOARD_SIZE - 1, col + 4); i++) {
if (board[row][i] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check vertical
count = 0;
for (int i = Math.max(0, row - 4); i <= Math.min(BOARD_SIZE - 1, row + 4); i++) {
if (board[i][col] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check diagonal (top-left to bottom-right)
count = 0;
for (int i = Math.max(row - 4, 0), j = Math.max(col - 4, 0); i <= Math.min(row + 4, BOARD_SIZE - 1) && j <= Math.min(col + 4, BOARD_SIZE - 1); i++, j++) {
if (board[i][j] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
// check diagonal (bottom-left to top-right)
count = 0;
for (int i = Math.min(row + 4, BOARD_SIZE - 1), j = Math.max(col - 4, 0); i >= Math.max(row - 4, 0) && j <= Math.min(col + 4, BOARD_SIZE - 1); i--, j++) {
if (board[i][j] == player) {
count++;
} else {
count = 0;
}
if (count == 5) {
return true;
}
}
return false;
}
private static boolean isBoardFull() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == EMPTY) {
return false;
}
}
}
return true;
}
}
```
这个程序实现了一个简单的五子棋游戏,玩家可以通过控制台输入行和列来下棋,程序会判断是否合法并更新棋盘,直到有一方获胜或棋盘满了为止。
相关推荐
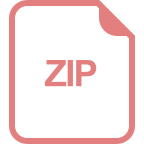
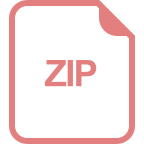
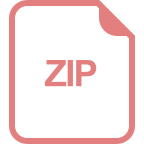













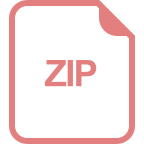