#include <stdio.h> #define MAXSIZE 100 typedef int KeyType; //根据需要设定数据类型 typedef struct { KeyType key; //关键字字段 }ElemType; typedef struct { ElemType r[MAXSIZE]; int length; // 表的长度 } SSTable; int BinSearch(SSTable t,int n,int k) //拆半查找算法 { /********** Begin **********/ /********** End **********/ } int main() { int i,j; int n,k; SSTable t; scanf("%d",&n); for (i=0;i<n;i++) scanf("%d",&t.r[i].key); scanf("%d",&k); j=BinSearch(t,n,k); if (j>=0 && j<n) printf("%d\n",t.r[j].key); else printf("-1\n"); }
时间: 2023-07-01 13:11:14 浏览: 164
好的,这段代码实现的是二分查找算法,以下是完整代码实现:
```c
#include <stdio.h>
#define MAXSIZE 100
typedef int KeyType; // 根据需要设定数据类型
typedef struct {
KeyType key; // 关键字字段
} ElemType;
typedef struct {
ElemType r[MAXSIZE];
int length; // 表的长度
} SSTable;
int BinSearch(SSTable t, int n, int k) // 拆半查找算法
{
int low = 0, high = n - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (t.r[mid].key == k) {
return mid;
} else if (t.r[mid].key > k) {
high = mid - 1;
} else {
low = mid + 1;
}
}
return -1;
}
int main()
{
int i, j;
int n, k;
SSTable t;
scanf("%d", &n);
for (i = 0; i < n; i++) {
scanf("%d", &t.r[i].key);
}
scanf("%d", &k);
j = BinSearch(t, n, k);
if (j >= 0 && j < n) {
printf("%d\n", t.r[j].key);
} else {
printf("-1\n");
}
return 0;
}
```
其中,`BinSearch` 函数使用了循环实现二分查找,即先在表中间位置确定一个中间值,将待查元素与中间值作比较,如果相等则返回该位置,如果待查元素小于中间值,则在左半部分继续查找,否则在右半部分继续查找,直到找到或者查找范围为空。
阅读全文
相关推荐













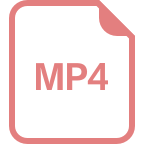
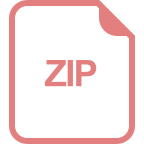
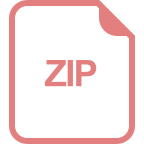