java代码 导出excel 设置表头序号,议题,种类 要求表头合并两行 用HSSFWorkbook写
时间: 2023-05-12 14:05:32 浏览: 65
以下是一个示例代码,可以实现导出excel并设置表头序号、议题、种类,并要求表头合并两行:
```
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
public class ExcelExporter {
public static void export(String[][] data) throws IOException {
HSSFWorkbook workbook = new HSSFWorkbook();
HSSFSheet sheet = workbook.createSheet("Sheet1");
// 设置表头样式
HSSFCellStyle headerStyle = workbook.createCellStyle();
headerStyle.setAlignment(HSSFCellStyle.ALIGN_CENTER);
// 设置表头内容
HSSFRow headerRow1 = sheet.createRow(0);
HSSFCell headerCell1 = headerRow1.createCell(0);
headerCell1.setCellValue("序号");
headerCell1.setCellStyle(headerStyle);
HSSFCell headerCell2 = headerRow1.createCell(1);
headerCell2.setCellValue("议题");
headerCell2.setCellStyle(headerStyle);
HSSFCell headerCell3 = headerRow1.createCell(2);
headerCell3.setCellValue("种类");
headerCell3.setCellStyle(headerStyle);
// 合并表头的两行
sheet.addMergedRegion(new CellRangeAddress(0, 1, 0, 0));
sheet.addMergedRegion(new CellRangeAddress(0, 1, 1, 1));
sheet.addMergedRegion(new CellRangeAddress(0, 1, 2, 2));
// 设置数据内容
for (int i = 0; i < data.length; i++) {
HSSFRow row = sheet.createRow(i + 2);
for (int j = 0; j < data[i].length; j++) {
HSSFCell cell = row.createCell(j);
cell.setCellValue(data[i][j]);
}
}
// 输出到文件
FileOutputStream fileOut = new FileOutputStream("output.xls");
workbook.write(fileOut);
fileOut.close();
}
}
```
你可以将数据传递给这个方法,然后它将生成一个名为 "output.xls" 的 Excel 文件,其中包含你提供的数据和所需的表头。
相关推荐
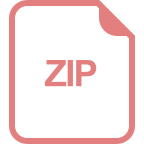
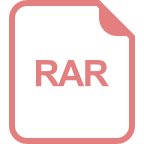
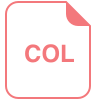
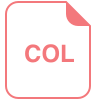
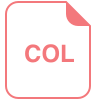
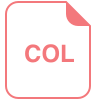
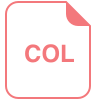









