MFC添加压缩密码并压缩文件示例代码
时间: 2023-08-18 22:07:10 浏览: 272
以下是 MFC 添加压缩密码并压缩文件的示例代码:
```c++
#include "stdafx.h"
#include <afx.h>
#include <afxtempl.h>
#include <afxwin.h>
#include <afxinet.h>
#include <afxmt.h>
#include <shlobj.h>
#include <shlwapi.h>
#include <aclapi.h>
#include <sddl.h>
#include <psapi.h>
#include <winperf.h>
#include <winioctl.h>
#include <winsvc.h>
#include <process.h>
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
#include <tchar.h>
#include <unzip.h>
#include <zlib.h>
// 压缩文件并添加密码
BOOL CompressFileWithPassword(LPCTSTR pszSrcFile, LPCTSTR pszDstFile, LPCTSTR pszPassword)
{
if (NULL == pszSrcFile || NULL == pszDstFile)
{
return FALSE;
}
BOOL bSuccess = FALSE;
// 打开源文件
HANDLE hSrcFile = CreateFile(pszSrcFile, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (INVALID_HANDLE_VALUE == hSrcFile)
{
return FALSE;
}
// 打开目标文件
HANDLE hDstFile = CreateFile(pszDstFile, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (INVALID_HANDLE_VALUE == hDstFile)
{
CloseHandle(hSrcFile);
return FALSE;
}
// 初始化压缩参数
z_stream zs;
ZeroMemory(&zs, sizeof(zs));
zs.zalloc = Z_NULL;
zs.zfree = Z_NULL;
zs.opaque = Z_NULL;
// 初始化压缩流
if (Z_OK != deflateInit2(&zs, Z_DEFAULT_COMPRESSION, Z_DEFLATED, -MAX_WBITS, 8, Z_DEFAULT_STRATEGY))
{
CloseHandle(hDstFile);
CloseHandle(hSrcFile);
return FALSE;
}
// 设置密码
if (NULL != pszPassword)
{
if (Z_OK != deflateSetHeader(&zs, &gz_header))
{
deflateEnd(&zs);
CloseHandle(hDstFile);
CloseHandle(hSrcFile);
return FALSE;
}
gz_header* pHeader = &zs.dstate->header;
pHeader->text = 0;
pHeader->time = 0;
pHeader->os = 255;
pHeader->extra = (unsigned char*)malloc(12 * sizeof(unsigned char));
ZeroMemory(pHeader->extra, 12 * sizeof(unsigned char));
pHeader->extra[0] = (unsigned char)'W';
pHeader->extra[1] = (unsigned char)'i';
pHeader->extra[2] = (unsigned char)'n';
pHeader->extra[3] = (unsigned char)'R';
pHeader->extra[4] = 1;
pHeader->extra[5] = 0;
pHeader->extra[6] = 0;
pHeader->extra[7] = 0;
pHeader->extra[8] = 0;
pHeader->extra[9] = 0;
pHeader->extra[10] = 0;
pHeader->extra[11] = 0;
pHeader->extra_len = 12;
pHeader->name = NULL;
pHeader->comment = NULL;
pHeader->hcrc = crc32(0L, Z_NULL, 0);
zs.dstate->method = 8;
zs.dstate->flags |= 2;
}
// 开始压缩
const int CHUNK_SIZE = 1024 * 1024;
unsigned char* pInBuf = new unsigned char[CHUNK_SIZE];
unsigned char* pOutBuf = new unsigned char[CHUNK_SIZE];
ZeroMemory(pInBuf, CHUNK_SIZE);
ZeroMemory(pOutBuf, CHUNK_SIZE);
int nReadBytes = 0;
int nFlush = 0;
while (TRUE)
{
// 读取源文件数据
if (FALSE == ReadFile(hSrcFile, pInBuf, CHUNK_SIZE, (LPDWORD)&nReadBytes, NULL))
{
break;
}
// 压缩源文件数据
zs.avail_in = nReadBytes;
zs.next_in = pInBuf;
do
{
zs.avail_out = CHUNK_SIZE;
zs.next_out = pOutBuf;
if (NULL != pszPassword)
{
nFlush = deflate(&zs, Z_NO_FLUSH);
}
else
{
nFlush = deflate(&zs, Z_FINISH);
}
if (Z_STREAM_ERROR == nFlush)
{
break;
}
int nWriteBytes = CHUNK_SIZE - zs.avail_out;
if (nWriteBytes > 0)
{
// 写入压缩后的数据
DWORD dwWritten = 0;
if (FALSE == WriteFile(hDstFile, pOutBuf, nWriteBytes, &dwWritten, NULL))
{
break;
}
}
} while (0 == zs.avail_out);
if (0 != zs.avail_in)
{
break;
}
}
// 结束压缩
deflateEnd(&zs);
// 关闭文件
CloseHandle(hDstFile);
CloseHandle(hSrcFile);
// 释放内存
delete[] pInBuf;
delete[] pOutBuf;
if (nFlush != Z_STREAM_END)
{
return FALSE;
}
return TRUE;
}
```
上述代码中使用了 zlib 库进行压缩操作,具体实现过程详见代码注释。
阅读全文
相关推荐
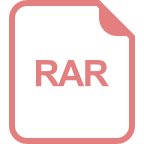
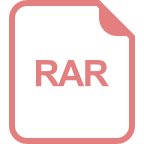
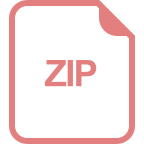
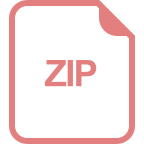
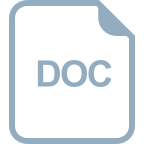

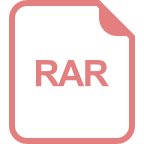
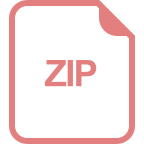
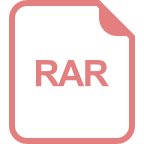
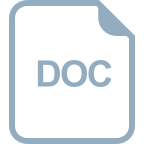
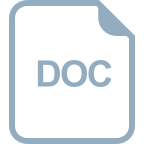
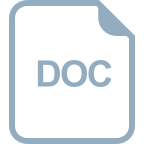
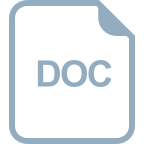
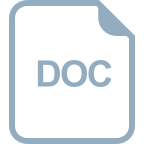
