建立三元组抽象数据类型定义的头文件,编程实现三元组的基本操作,输出任意三元组的最大值和最小值。
时间: 2023-04-25 11:05:36 浏览: 84
以下是三元组抽象数据类型定义的头文件:
```
#ifndef TRIPLE_H
#define TRIPLE_H
typedef struct {
int first;
int second;
int third;
} Triple;
Triple createTriple(int first, int second, int third);
int getFirst(Triple t);
int getSecond(Triple t);
int getThird(Triple t);
void setFirst(Triple *t, int first);
void setSecond(Triple *t, int second);
void setThird(Triple *t, int third);
int getMax(Triple t);
int getMin(Triple t);
#endif
```
以下是三元组的基本操作的实现:
```
#include "triple.h"
Triple createTriple(int first, int second, int third) {
Triple t;
t.first = first;
t.second = second;
t.third = third;
return t;
}
int getFirst(Triple t) {
return t.first;
}
int getSecond(Triple t) {
return t.second;
}
int getThird(Triple t) {
return t.third;
}
void setFirst(Triple *t, int first) {
t->first = first;
}
void setSecond(Triple *t, int second) {
t->second = second;
}
void setThird(Triple *t, int third) {
t->third = third;
}
int getMax(Triple t) {
int max = t.first;
if (t.second > max) {
max = t.second;
}
if (t.third > max) {
max = t.third;
}
return max;
}
int getMin(Triple t) {
int min = t.first;
if (t.second < min) {
min = t.second;
}
if (t.third < min) {
min = t.third;
}
return min;
}
```
以下是输出任意三元组的最大值和最小值的示例代码:
```
#include <stdio.h>
#include "triple.h"
int main() {
Triple t = createTriple(3, 7, 2);
printf("Max: %d\n", getMax(t));
printf("Min: %d\n", getMin(t));
return ;
}
```
相关推荐
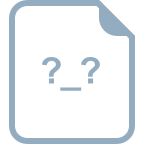
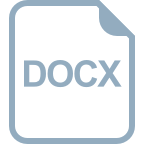














