opencv python形状和颜色识别
时间: 2023-10-12 13:06:04 浏览: 65
要实现形状和颜色识别,可以使用OpenCV和Python。下面是一个示例代码,可以识别三种形状(圆形、矩形和三角形)和三种颜色(红色、绿色和蓝色):
```python
import cv2
import numpy as np
# 定义颜色范围
lower_red = np.array([0, 100, 100])
upper_red = np.array([10, 255, 255])
lower_green = np.array([50, 100, 100])
upper_green = np.array([70, 255, 255])
lower_blue = np.array([110, 100, 100])
upper_blue = np.array([130, 255, 255])
# 读取图像
img = cv2.imread('shapes.jpg')
# 将图像转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对图像进行模糊处理
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# 边缘检测
edges = cv2.Canny(blur, 50, 150)
# 查找轮廓
contours, hierarchy = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 定义空列表存储结果
shapes = []
# 遍历每个轮廓
for cnt in contours:
# 计算轮廓周长
perimeter = cv2.arcLength(cnt, True)
# 进行轮廓近似
approx = cv2.approxPolyDP(cnt, 0.04 * perimeter, True)
# 计算轮廓面积
area = cv2.contourArea(cnt)
# 计算轮廓的形状
if len(approx) == 3:
shape = "triangle"
elif len(approx) == 4:
x, y, w, h = cv2.boundingRect(cnt)
aspect_ratio = float(w) / h
if aspect_ratio >= 0.95 and aspect_ratio <= 1.05:
shape = "square"
else:
shape = "rectangle"
else:
shape = "circle"
# 计算轮廓的颜色
mask = np.zeros(img.shape[:2], dtype=np.uint8)
cv2.drawContours(mask, [cnt], -1, 255, -1)
mean_color = cv2.mean(img, mask=mask)[:3]
color = None
if mean_color[2] > mean_color[1] and mean_color[2] > mean_color[0]:
color = "red"
elif mean_color[1] > mean_color[2] and mean_color[1] > mean_color[0]:
color = "green"
else:
color = "blue"
# 将结果添加到列表中
shapes.append((shape, color))
# 在图像上绘制识别结果
font = cv2.FONT_HERSHEY_SIMPLEX
for i, (shape, color) in enumerate(shapes):
x, y = contours[i][0][0]
cv2.putText(img, shape + " " + color, (x, y), font, 1, (255, 255, 255), 2, cv2.LINE_AA)
cv2.drawContours(img, [contours[i]], -1, (0, 255, 0), 2)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们首先定义了三种颜色的范围,然后读取图像并将其转换为灰度图像。接下来,我们对图像进行模糊处理和边缘检测,并查找轮廓。对于每个轮廓,我们计算其周长和面积,并使用轮廓近似算法确定其形状。我们还使用一个掩膜来计算轮廓的颜色。
最后,我们将识别结果绘制在图像上,并显示结果。
相关推荐
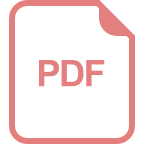
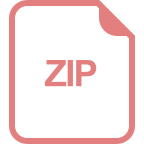
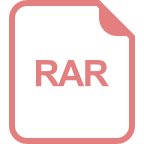














