SSM框架web开发代码
时间: 2023-08-13 07:15:38 浏览: 41
SSM(Spring + SpringMVC + MyBatis)是一种常用的 Java Web 开发框架组合,下面是一个简单的 SSM 框架的示例代码,用于实现一个简单的增删改查的学生信息管理系统:
首先是 `Student` 实体类:
```java
public class Student {
private Integer id;
private String name;
private Integer age;
private String gender;
private String major;
// 省略 getter 和 setter 方法
}
```
接下来是 `StudentMapper` 接口,用于定义增删改查的方法:
```java
public interface StudentMapper {
List<Student> getAllStudents();
Student getStudentById(Integer id);
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(Integer id);
}
```
然后是 `StudentMapper.xml` 文件,用于实现这些方法:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.StudentMapper">
<resultMap id="StudentResultMap" type="com.example.entity.Student">
<id column="id" property="id" />
<result column="name" property="name" />
<result column="age" property="age" />
<result column="gender" property="gender" />
<result column="major" property="major" />
</resultMap>
<select id="getAllStudents" resultMap="StudentResultMap">
SELECT * FROM student;
</select>
<select id="getStudentById" resultMap="StudentResultMap">
SELECT * FROM student WHERE id=#{id};
</select>
<insert id="addStudent">
INSERT INTO student (name, age, gender, major) VALUES (#{name}, #{age}, #{gender}, #{major});
</insert>
<update id="updateStudent">
UPDATE student SET name=#{name}, age=#{age}, gender=#{gender}, major=#{major} WHERE id=#{id};
</update>
<delete id="deleteStudent">
DELETE FROM student WHERE id=#{id};
</delete>
</mapper>
```
然后是 `StudentService` 接口,用于定义具体的增删改查操作:
```java
public interface StudentService {
List<Student> getAllStudents();
Student getStudentById(Integer id);
void addStudent(Student student);
void updateStudent(Student student);
void deleteStudent(Integer id);
}
```
接下来是 `StudentServiceImpl` 类,用于实现这些操作:
```java
@Service
public class StudentServiceImpl implements StudentService {
@Autowired
private StudentMapper studentMapper;
@Override
public List<Student> getAllStudents() {
return studentMapper.getAllStudents();
}
@Override
public Student getStudentById(Integer id) {
return studentMapper.getStudentById(id);
}
@Override
public void addStudent(Student student) {
studentMapper.addStudent(student);
}
@Override
public void updateStudent(Student student) {
studentMapper.updateStudent(student);
}
@Override
public void deleteStudent(Integer id) {
studentMapper.deleteStudent(id);
}
}
```
最后是 `StudentController` 类,用于处理 HTTP 请求和响应:
```java
@Controller
@RequestMapping("/student")
public class StudentController {
@Autowired
private StudentService studentService;
@RequestMapping("/list")
public String list(Model model) {
List<Student> students = studentService.getAllStudents();
model.addAttribute("students", students);
return "list";
}
@RequestMapping("/add")
public String add() {
return "add";
}
@RequestMapping("/doAdd")
public String doAdd(Student student) {
studentService.addStudent(student);
return "redirect:/student/list";
}
@RequestMapping("/edit")
public String edit(Integer id, Model model) {
Student student = studentService.getStudentById(id);
model.addAttribute("student", student);
return "edit";
}
@RequestMapping("/doEdit")
public String doEdit(Student student) {
studentService.updateStudent(student);
return "redirect:/student/list";
}
@RequestMapping("/delete")
public String delete(Integer id) {
studentService.deleteStudent(id);
return "redirect:/student/list";
}
}
```
在上面的代码中,`@Controller` 注解表示这是一个控制器类,`@RequestMapping` 注解用于映射 HTTP 请求路径。`Model` 参数用于向视图传递数据。`redirect:/student/list` 表示重定向到 `/student/list` 路径。
最后,在 `src/main/resources` 目录下创建 `applicationContext.xml` 文件,配置 Spring、MyBatis 和数据库连接信息:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.3.xsd">
<context:component-scan base-package="com.example" />
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.example.mapper" />
</bean>
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/test" />
<property name="username" value="root" />
<property name="password" value="" />
</bean>
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="typeAliasesPackage" value="com.example.entity" />
<property name="mapperLocations" value="classpath:com/example/mapper/*.xml" />
</bean>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<tx:annotation-driven />
</beans>
```
这里使用的是 MySQL 数据库,修改 `url`、`username` 和 `password` 属性以适应你的环境。
以上就是一个简单的 SSM 框架的示例代码,它实现了一个简单的增删改查的学生信息管理系统。
相关推荐
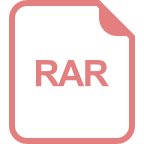














