public class A { public static void main(String[] args) { int a=6; int b=4; int c=4; if(a==++b+1)a>>=(b-c); System.out.println(a); } }
时间: 2024-03-15 09:41:39 浏览: 20
根据这段 Java 代码,输出结果应该是 3。代码中定义了三个整型变量 a、b、c,分别初始化为 6、4、4。在 if 语句中,++b 先将变量 b 的值加 1,变成了 5,然后再加 1,变成了 6。因此,if 语句的条件为 a==7,这个条件不成立,所以 if 语句的代码块不会执行。
因此,最后输出变量 a 的值,即 6,因为没有对变量 a 进行任何修改。
相关问题
public class test { public static void main(String args[]) { int a=10; int b; int c; if(a>50) { b=9; } c=b+a; } }
在你提供的代码中,存在一个问题,即变量b和c没有初始化。在Java中,局部变量必须在使用之前进行初始化。因此,在你的代码中,你需要确保变量b和c都有合适的初始值。
以下是修正后的代码:
```java
public class Test {
public static void main(String args[]) {
int a = 10;
int b = 0;
int c = 0;
if (a > 50) {
b = 9;
}
c = b + a;
System.out.println("c = " + c);
}
}
```
在这个修正后的代码中,我对变量b和c进行了初始化,以确保在使用之前都有合适的值。最后,我还添加了一行代码,用于打印变量c的值。
class demo{public static void main(int x=div(1,2}
在这个问题里,需要对Java语言的语法和调用函数的方式有一定的了解。
首先,在Java中,main方法的参数是一个字符串数组,而不是一个整数。另外,在方法参数的定义中不可以给参数一个默认的初始值。
在这个例子中,如果要调用一个div函数并将结果赋值给x,首先需要定义一个div函数。假设div函数是一个可以接受两个整数参数并返回它们相除的函数,那么它的定义可能是这样的:
public static int div(int a, int b){
return a / b;
}
然后,在main方法中调用div函数,并将结果赋值给x,可以这样写:
public static void main(String[] args){
int x = div(1, 2);
}
上面的代码中,main方法接受一个字符串数组参数args。在方法体内部,使用div(1, 2)的结果赋值给x。这样x的值就是1除以2的结果,即0。
综上所述,能正确执行的代码应该是:
public class demo{
public static int div(int a, int b){
return a / b;
}
public static void main(String[] args){
int x = div(1, 2);
}
}
希望以上内容能够解答您的问题。如有其他疑问,欢迎继续询问。
相关推荐
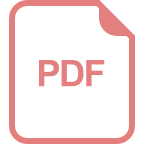
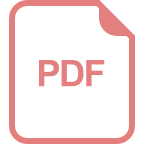
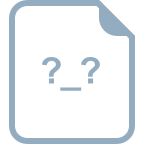












