从键盘输入一个年份year和月份month,输出该月有多少天(考虑闰年)。 提示:如果年份能被400整除,则它是闰年;如果能被4整除,而不能被100整除,则也是闰年,否则不是闰年。
时间: 2023-05-31 14:18:35 浏览: 290
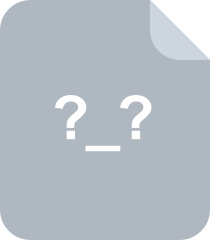
python 输入年份 如果是闰年输出True 否则输出False 示例

### 回答1:
请使用以下代码输入一个年份year和月份month,输出该月有多少天(考虑闰年):
```
year = int(input("请输入年份: "))
month = int(input("请输入月份: "))
if month in (1, 3, 5, 7, 8, 10, 12):
days = 31
elif month == 2:
if (year % 400 == 0) or (year % 4 == 0 and year % 100 != 0):
days = 29
else:
days = 28
else:
days = 30
print(f'{month}月有{days}天')
```
这段代码首先会询问用户输入年份和月份,然后使用 if-elif-else 结构来检查该月的天数。如果月份是1,3,5,7,8,10,12之一,则天数为31。如果月份是2月,则需要检查该年份是否为闰年,如果是闰年,则天数为29,否则天数为28。对于其他月份,天数为30.最后输出该月有多少天.
### 回答2:
这道题目要求我们根据用户输入的年份和月份,判断该月份有多少天,需要考虑闰年的因素。
首先我们需要判断年份是否为闰年。如果年份能被400整除,则它是闰年;如果能被4整除,而不能被100整除,则也是闰年,否则不是闰年。
判断完闰年后,我们可以根据月份来计算出该月有多少天。对于大月份(1、3、5、7、8、10、12月),有31天;对于小月份(4、6、9、11月),有30天;对于2月份,需要根据闰年与否来计算。如果是闰年,则有29天,否则只有28天。
因此,我们可以写出如下代码:
```
#include <stdio.h>
int main()
{
int year, month, days;
printf("请输入年份和月份:\n");
scanf("%d%d", &year, &month);
// 判断是否为闰年
if((year % 400 == 0) || (year % 4 == 0 && year % 100 != 0))
{
// 闰年
if(month == 2)
{
days = 29;
}
else if(month == 4 || month == 6 || month == 9 || month == 11)
{
days = 30;
}
else
{
days = 31;
}
}
else
{
// 不是闰年
if(month == 2)
{
days = 28;
}
else if(month == 4 || month == 6 || month == 9 || month == 11)
{
days = 30;
}
else
{
days = 31;
}
}
printf("%d年%d月有%d天\n", year, month, days);
return 0;
}
```
以上就是完整的代码,根据用户输入的年份和月份,我们先判断闰年的情况,再根据月份来计算出该月有多少天,最后输出结果给用户。
总体来说,这道题目难度不大,考察了我们的逻辑思维以及对条件语句和运算符的掌握程度。在编写代码时,我们需要考虑各种情况,把所有可能的情况都覆盖到,以确保程序的正确性。
### 回答3:
这道题需要考虑到闰年的情况。闰年是指四年一闰,百年不闰,四百年再闰。因此,要判断一个年份是否为闰年,需要满足两个条件:能被4整除但不能被100整除,或者能被400整除。接下来就是判断每个月的天数了。
对于大月,如1、3、5、7、8、10、12月,它们都有31天;而对于小月,如4、6、9、11月,它们只有30天。对于2月,它有28天,闰年的2月有29天。
因此,可以先通过判断年份是否为闰年来确定2月份的天数,然后再判断其他月份即可。
下面是一个简单的代码实现:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
# 判断闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
if month == 2:
days = 29
elif month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
else:
days = 30
else:
if month == 2:
days = 28
elif month in [1, 3, 5, 7, 8, 10, 12]:
days = 31
else:
days = 30
print("{}年{}月有{}天。".format(year, month, days))
```
通过键盘输入一个年份和月份后,便可输出该月的天数。
阅读全文
相关推荐













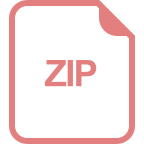