帮我写一个c++的N皇后问题的回溯算法
时间: 2023-08-28 10:16:51 浏览: 49
好的,下面是一个用C++实现的N皇后问题的回溯算法:
```
#include <iostream>
#include <vector>
using namespace std;
bool is_valid(vector<int>& queens, int row, int col) {
for (int i = 0; i < row; i++) {
if (queens[i] == col || abs(queens[i] - col) == abs(i - row)) {
return false;
}
}
return true;
}
void n_queens(vector<int>& queens, int row, int n, int& count) {
if (row == n) {
count++;
return;
}
for (int col = 0; col < n; col++) {
if (is_valid(queens, row, col)) {
queens[row] = col;
n_queens(queens, row + 1, n, count);
}
}
}
int main() {
int n = 8;
int count = 0;
vector<int> queens(n);
n_queens(queens, 0, n, count);
cout << "The number of solutions for " << n << " queens is " << count << endl;
return 0;
}
```
在这个实现中,我们使用一个`vector<int>`来存储皇后的位置,其中`queens[i]`表示第i行皇后所在的列数。`is_valid`函数用来检查当前位置是否合法,如果当前位置和之前的皇后有冲突,则返回false。`n_queens`函数用来递归地搜索解空间,当搜索到第n行时,说明找到了一组合法的解,将计数器加1即可。
在主函数中,我们调用`n_queens`函数来搜索解空间,然后输出结果。
需要注意的是,这个算法只能求解N皇后问题的解的数量,如果需要求出具体的解,需要做一些修改。
相关推荐
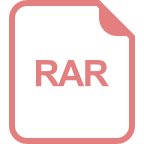
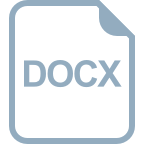
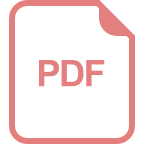














