python使用d2l包,通过线性回归模拟sinx函数
时间: 2024-05-20 16:16:47 浏览: 174
以下是使用d2l包在Python中模拟sinx函数的线性回归代码:
```
import numpy as np
import matplotlib.pyplot as plt
import d2l
# 生成数据集
n = 1000
X = np.random.uniform(-np.pi, np.pi, size=(n, 1))
y = np.sin(X) + np.random.normal(scale=0.1, size=(n, 1))
# 绘制数据集
d2l.set_figsize()
plt.scatter(X, y, 1)
plt.show()
# 数据集读取器
def data_iter(batch_size):
n = len(X)
indices = list(range(n))
np.random.shuffle(indices)
for i in range(0, n, batch_size):
indexs = np.array(indices[i: min(i+batch_size, n)])
yield X[indexs], y[indexs]
# 初始化模型参数
w = np.random.normal(scale=0.01, size=(1, 1))
b = np.zeros((1, 1))
# 定义模型
def linreg(X, w, b):
return np.dot(X, w) + b
# 定义损失函数
def squared_loss(y_hat, y):
return (y_hat - y.reshape(y_hat.shape)) ** 2 / 2
# 定义优化算法
def sgd(params, lr, batch_size):
for param in params:
param -= lr * param.grad / batch_size
param.grad.zeros_()
# 训练模型
lr = 0.03
num_epochs = 3
net = linreg
loss = squared_loss
batch_size = 10
for epoch in range(num_epochs):
for X, y in data_iter(batch_size):
l = loss(net(X, w, b), y)
l.sum().backward()
sgd([w, b], lr, batch_size)
train_l = loss(net(X, w, b), y)
print('epoch %d, loss %.4f' % (epoch + 1, train_l.mean()))
# 绘制拟合曲线
d2l.set_figsize()
plt.scatter(X, y, 1)
plt.plot(X, net(X, w, b), color='red')
plt.show()
```
在运行该代码之后,将得到一个类似于下图的拟合曲线:

从图中可以看出,拟合曲线与sinx函数相似,但并不完全一致。这是因为添加了高斯噪声的数据集是不完美的,因此无法通过线性回归精确地还原出原始的sinx函数。
阅读全文
相关推荐
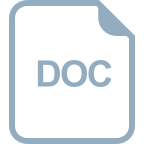
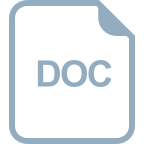
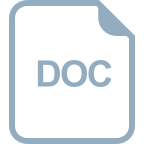
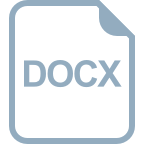
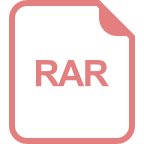
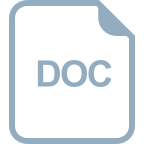
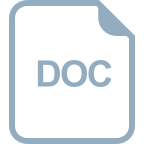
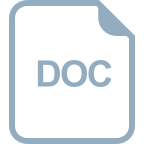








