CCPP路径规划遗传算法代码
时间: 2023-03-20 15:01:05 浏览: 110
以下是使用遗传算法解决CCPP(Combined Cycle Power Plant)路径规划问题的Python代码:
```python
import random
# Constants
POP_SIZE = 50 # Population size
GENE_SIZE = 10 # Number of genes in each chromosome
MUTATION_RATE = 0.01 # Mutation rate
ELITE_RATE = 0.1 # Elite rate
GENERATIONS = 1000 # Number of generations
# Fitness function
def fitness(chromosome):
# Calculate the total distance
distance = 0
for i in range(GENE_SIZE - 1):
x1, y1 = chromosome[i]
x2, y2 = chromosome[i+1]
distance += ((x2-x1)**2 + (y2-y1)**2) ** 0.5
return 1 / distance
# Selection function
def selection(population):
# Sort the population by fitness
population = sorted(population, key=lambda x: fitness(x), reverse=True)
# Calculate the elite size
elite_size = int(ELITE_RATE * POP_SIZE)
# Select the elite chromosomes
elite = population[:elite_size]
# Select the remaining chromosomes using roulette wheel selection
fitness_sum = sum([fitness(chromosome) for chromosome in population])
roulette_wheel = [fitness(chromosome) / fitness_sum for chromosome in population]
selection = random.choices(population, weights=roulette_wheel, k=POP_SIZE-elite_size)
return elite + selection
# Crossover function
def crossover(parent1, parent2):
# Select a random crossover point
crossover_point = random.randint(1, GENE_SIZE-1)
# Create the offspring chromosomes
offspring1 = parent1[:crossover_point] + parent2[crossover_point:]
offspring2 = parent2[:crossover_point] + parent1[crossover_point:]
return offspring1, offspring2
# Mutation function
def mutation(chromosome):
# Iterate over each gene
for i in range(GENE_SIZE):
# Apply the mutation rate
if random.random() < MUTATION_RATE:
# Swap the gene with another random gene
j = random.randint(0, GENE_SIZE-1)
chromosome[i], chromosome[j] = chromosome[j], chromosome[i]
return chromosome
# Initialization
population = [[(random.uniform(0, 1), random.uniform(0, 1))) for j in range(GENE_SIZE)] for i in range(POP_SIZE)]
# Main loop
for generation in range(GENERATIONS):
# Selection
population = selection(population)
# Crossover
offspring = []
for i in range(0, POP_SIZE-1, 2):
offspring1, offspring2 = crossover(population[i], population[i+1])
offspring.append(offspring1)
offspring.append(offspring2)
# Mutation
population = [mutation(chromosome) for chromosome in offspring]
# Print the best fitness
best_fitness = fitness(population[0])
print("Generation:", generation+1, "Best fitness:", best_fitness)
```
该代码使用遗传算法解决CCPP路径规划问题。在该问题中,我们需要找到一条连接多个点的路径,使得路径总长度最小。该代码的实现包括以下主要步骤:
1. 初始化种群:使用随机数生成器生成一组随机的染色体,每个染色体
相关推荐
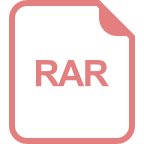

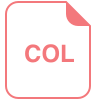

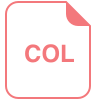










