用stm32实现从串口1接收由计算机发送过来的6个字节的数据串,其中,最后一个字节是前面5个字节的异或值(称为校验值)。在程序中进行校验,若计算的校验值和收到的校验值相同,则发回1;否则,发回0。通信参数:9600,n,8,1
时间: 2023-12-18 11:05:14 浏览: 23
下是一个简单的实现,首先需要开启串口1的接收中断,并在中断中将接收到的数据存储到一个数组中。当接收的字节数达到6个时,进行校验,并将结果通过串口1发送回计算机。
```c
#include "stm32f10x.h"
uint8_t rx_buf[6];
uint8_t rx_cnt = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
rx_buf[rx_cnt] = USART_ReceiveData(USART1);
rx_cnt++;
if (rx_cnt == 6)
{
uint8_t check = 0;
for (int i = 0; i < 5; i++)
check ^= rx_buf[i];
if (check == rx_buf[5])
USART_SendData(USART1, 0x31); // 发送1
else
USART_SendData(USART1, 0x30); // 发送0
rx_cnt = 0;
}
}
}
int main(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// 使能USART1和GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1 Tx (PA9) 为复用推挽输出
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1 Rx (PA10) 为浮空输入
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 配置USART1中断
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 1;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// 使能USART1
USART_Cmd(USART1, ENABLE);
while (1)
{
// 等待中断处理数据
}
}
```
相关推荐
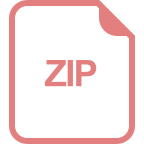
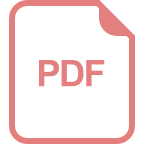














