写一个stm32c8t6 的串口1 接收和发送的函数
时间: 2024-04-13 09:30:11 浏览: 19
下面是一个示例代码,用于在STM32C8T6上编写串口1的接收和发送函数:
```c
#include "stm32f10x.h"
#define BUFFER_SIZE 128
volatile uint8_t rxBuffer[BUFFER_SIZE];
volatile uint8_t rxIndex = 0;
void USART1_IRQHandler(void) {
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) { // 检查是否接收到数据
uint8_t data = USART_ReceiveData(USART1); // 读取接收到的数据
if (rxIndex < BUFFER_SIZE) {
rxBuffer[rxIndex++] = data; // 存储数据到接收缓冲区
}
}
}
void USART1_SendByte(uint8_t data) {
while (USART_GetFlagStatus(USART1, USART_FLAG_TC) == RESET); // 等待发送缓冲区为空
USART_SendData(USART1, data); // 发送数据
}
void USART1_SendString(const char* str) {
while (*str) {
USART1_SendByte(*str++);
}
}
int main(void) {
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// 使能串口1时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// 使能GPIOA时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置PA9作为USART1的TX引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置PA10作为USART1的RX引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 配置USART1接收中断
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// 使能USART1接收中断
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// 使能USART1
USART_Cmd(USART1, ENABLE);
while (1) {
// 在这里可以处理接收到的数据
if (rxIndex > 0) {
// 处理接收缓冲区中的数据
// ...
rxIndex = 0; // 清空接收缓冲区索引
}
// 发送数据示例
USART1_SendString("Hello, World!\r\n");
}
}
```
以上代码演示了如何在STM32C8T6上配置和使用USART1进行串口接收和发送。在USART1的中断处理函数中(`USART1_IRQHandler`),通过检查`USART_GetITStatus(USART1, USART_IT_RXNE)`来判断是否接收到数据。如果接收到数据,使用`USART_ReceiveData(USART1)`读取接收到的数据,并将其存储到接收缓冲区`rxBuffer`中。
在主函数中,可以通过处理接收缓冲区的数据来进行相应的操作。请注意,这只是一个示例,你可以根据自己的需求进行进一步的处理。同时,`USART1_SendByte()`函数用于发送单个字节数据,`USART1_SendString()`函数用于发送字符串数据。在示例中,发送了"Hello, World!\r\n"字符串。记得根据实际情况修改波特率和其他参数。
相关推荐
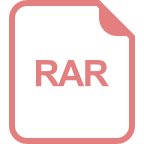
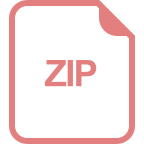














