设计一个有弹窗的Java英汉互译词典包含添加,删除,查找功能
时间: 2024-03-11 09:51:06 浏览: 26
好的,我可以帮你设计一个简单的Java英汉互译词典,具备添加、删除和查找功能,并包含弹窗。
首先,我们需要一个词典类 `Dictionary`,它可以保存英文单词和对应的中文翻译。我们可以使用一个 `HashMap` 来保存这些信息。
```java
import java.util.HashMap;
public class Dictionary {
private HashMap<String, String> dict;
public Dictionary() {
dict = new HashMap<>();
}
public void addWord(String word, String translation) {
dict.put(word, translation);
}
public void removeWord(String word) {
dict.remove(word);
}
public String findTranslation(String word) {
return dict.get(word);
}
}
```
接下来,我们需要一个窗口类 `DictionaryWindow`,它包含了添加、删除和查找功能,并且可以弹出相应的窗口进行交互。我们可以使用 `JFrame` 和 `JOptionPane` 来实现这些功能。
```java
import javax.swing.JFrame;
import javax.swing.JOptionPane;
public class DictionaryWindow extends JFrame {
private Dictionary dict;
public DictionaryWindow() {
dict = new Dictionary();
setTitle("英汉互译词典");
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void addWord() {
String word = JOptionPane.showInputDialog(this, "请输入英文单词:");
String translation = JOptionPane.showInputDialog(this, "请输入中文翻译:");
dict.addWord(word, translation);
JOptionPane.showMessageDialog(this, "添加成功!");
}
public void removeWord() {
String word = JOptionPane.showInputDialog(this, "请输入要删除的英文单词:");
dict.removeWord(word);
JOptionPane.showMessageDialog(this, "删除成功!");
}
public void findTranslation() {
String word = JOptionPane.showInputDialog(this, "请输入要查找的英文单词:");
String translation = dict.findTranslation(word);
if (translation != null) {
JOptionPane.showMessageDialog(this, word + " 的中文翻译是:" + translation);
} else {
JOptionPane.showMessageDialog(this, "抱歉,没有找到 " + word + " 的翻译。");
}
}
}
```
最后,我们可以在 `main` 方法中创建一个 `DictionaryWindow` 对象,并添加相应的按钮来调用上述方法,实现整个词典的交互过程。
```java
public class Main {
public static void main(String[] args) {
DictionaryWindow window = new DictionaryWindow();
JButton addButton = new JButton("添加单词");
addButton.addActionListener(e -> window.addWord());
JButton removeButton = new JButton("删除单词");
removeButton.addActionListener(e -> window.removeWord());
JButton findButton = new JButton("查找单词");
findButton.addActionListener(e -> window.findTranslation());
JPanel panel = new JPanel();
panel.add(addButton);
panel.add(removeButton);
panel.add(findButton);
window.add(panel);
}
}
```
这样,我们就完成了一个简单的Java英汉互译词典,包含了添加、删除和查找功能,并包含弹窗。
相关推荐
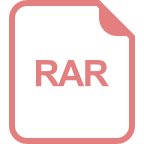














