用Java设计一个有弹窗的英汉词典,包含添加,删除和查找功能
时间: 2024-03-17 17:45:58 浏览: 15
好的,我可以为您提供一个简单的Java词典程序。
首先,您需要创建一个界面,使用Swing库中的JFrame和JPanel。接下来,您可以使用JTextField实现一个搜索框和一个添加/删除按钮。搜索框将用于输入英文单词,添加/删除按钮将用于添加或删除单词和其相应的中文翻译。最后,您可以使用JOptionPane类来实现弹窗功能。
以下是一个简单的代码示例:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.HashMap;
import java.util.Map;
public class Dictionary extends JFrame implements ActionListener {
private JTextField textField;
private JButton addButton, deleteButton, searchButton;
private JTextArea textArea;
private Map<String, String> dictionary;
public Dictionary() {
super("英汉词典");
dictionary = new HashMap<>();
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(2, 1));
JPanel searchPanel = new JPanel();
searchPanel.setLayout(new GridLayout(1, 3));
textField = new JTextField();
searchButton = new JButton("搜索");
searchButton.addActionListener(this);
searchPanel.add(textField);
searchPanel.add(searchButton);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(1, 2));
addButton = new JButton("添加单词");
addButton.addActionListener(this);
deleteButton = new JButton("删除单词");
deleteButton.addActionListener(this);
buttonPanel.add(addButton);
buttonPanel.add(deleteButton);
panel.add(searchPanel);
panel.add(buttonPanel);
textArea = new JTextArea();
textArea.setEditable(false);
add(panel, BorderLayout.NORTH);
add(new JScrollPane(textArea), BorderLayout.CENTER);
setSize(400, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == searchButton) {
String word = textField.getText().trim();
if (dictionary.containsKey(word)) {
JOptionPane.showMessageDialog(this, dictionary.get(word));
} else {
JOptionPane.showMessageDialog(this, "未找到该单词");
}
} else if (e.getSource() == addButton) {
String word = JOptionPane.showInputDialog(this, "请输入要添加的单词");
if (word != null && !word.trim().isEmpty()) {
String translation = JOptionPane.showInputDialog(this, "请输入单词的中文翻译");
dictionary.put(word.trim(), translation.trim());
JOptionPane.showMessageDialog(this, "添加成功");
}
} else if (e.getSource() == deleteButton) {
String word = JOptionPane.showInputDialog(this, "请输入要删除的单词");
if (word != null && !word.trim().isEmpty()) {
if (dictionary.containsKey(word.trim())) {
dictionary.remove(word.trim());
JOptionPane.showMessageDialog(this, "删除成功");
} else {
JOptionPane.showMessageDialog(this, "未找到该单词");
}
}
}
}
public static void main(String[] args) {
new Dictionary();
}
}
```
在这个示例中,我们定义了一个名为Dictionary的类,它继承自JFrame,并实现了ActionListener接口以便处理按钮事件。我们使用HashMap来保存单词和翻译的对应关系,使用JOptionPane来实现弹窗功能。在actionPerformed方法中,我们根据按钮的不同实现了不同的功能,例如搜索、添加和删除单词。最后,在main方法中创建了一个Dictionary对象,以启动程序。
希望这个示例能够帮助您实现您的英汉词典程序。
相关推荐
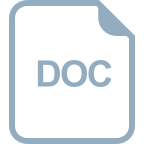














