#include <stdio.h> #include <string.h> #include <stdlib.h> // 定义结构体类型 struct Student { char id[10]; // 学号 char name[20]; // 姓名 char gender[10]; // 性别 int chinese; // 语文成绩 int math; // 数学成绩 int english; // 英语成绩 }; // 初始化几个学生的基本信息 struct Student students[] = { {"1001", "张三", "Male", 80, 90, 85}, {"1002", "李四", "Female", 75, 85, 90}, {"1003", "王五", "Male", 90, 80, 95}, {"1004", "赵六", "Female", 85, 95, 80}, {"1005", "钱七", "Male", 95, 85, 90} }; int count = 5; // 学生数量//添加一个学生信息 void add_student(struct Student* new_student) { int size = sizeof(students) / sizeof(students[0]); struct Student* temp = (struct Student*)realloc(students, (size + 1) * sizeof(struct Student)); if (temp != NULL) { students = temp; // 更新 students 指针指向的内存 for (int i = 0; i < 5; i++) { students[i] = Student(); // 初始化每个元素 } memcpy(&students[size], new_student, sizeof(struct Student)); printf("添加成功!\n"); } else { printf("添加失败!\n"); } }修改这段代码
时间: 2024-01-28 20:03:51 浏览: 26
这段代码的功能是定义了一个学生结构体类型,初始化了几个学生的基本信息,并提供了一个添加学生信息的函数。在添加学生信息的函数中,使用realloc函数动态扩展了存储学生信息的数组,然后将新的学生信息拷贝到数组末尾。
以下是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
struct Student {
char id[10];
char name[20];
char gender[10];
int chinese;
int math;
int english;
};
// 初始化几个学生的基本信息
struct Student students[] = {
{"1001", "张三", "Male", 80, 90, 85},
{"1002", "李四", "Female", 75, 85, 90},
{"1003", "王五", "Male", 90, 80, 95},
{"1004", "赵六", "Female", 85, 95, 80},
{"1005", "钱七", "Male", 95, 85, 90}
};
int count = 5; // 学生数量
// 添加一个学生信息
void add_student(struct Student* new_student) {
int size = sizeof(students) / sizeof(students[0]);
struct Student* temp = (struct Student*)realloc(students, (size + 1) * sizeof(struct Student));
if (temp != NULL) {
students = temp; // 更新 students 指针指向的内存
memcpy(&students[size], new_student, sizeof(struct Student));
count++;
printf("添加成功!\n");
}
else {
printf("添加失败!\n");
}
}
int main() {
// 添加一个新的学生
struct Student new_student = {"1006", "孙八", "Male", 88, 77, 66};
add_student(&new_student);
// 输出所有学生信息
printf("学号\t姓名\t性别\t语文\t数学\t英语\n");
for (int i = 0; i < count; i++) {
printf("%s\t%s\t%s\t%d\t%d\t%d\n", students[i].id, students[i].name, students[i].gender, students[i].chinese, students[i].math, students[i].english);
}
return 0;
}
```
修改的内容:
1. 在添加学生信息的函数中,去掉了初始化每个元素的循环,因为realloc函数已经为新的内存分配了空间并初始化为0。
2. 在添加学生信息的函数中,将学生数量count增加1。
3. 在main函数中添加一个新的学生,并且输出所有学生的信息。
相关推荐
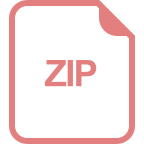
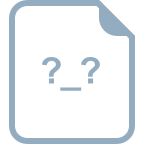
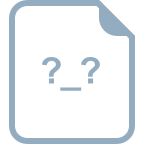













