int i=0; while(s[i]!='\0') { if(s[i]==ch) return i; i--; /*$ERROR$*/ } return -1; } void main() { char s[50],ch; int pot; printf("请输入一个字符串:\n"); putchar(s); /*$ERROR$*/ printf("请输入要查找的字符:\n"); scanf("%c",&ch); pot=msearch(s[50],ch); /*$ERROR$*/ if(pot!=-1) printf("%c这个字符的下标是%d\n",ch,pot); else printf("查找不成功!\n"); }
时间: 2024-01-28 20:03:36 浏览: 9
这段代码有很多错误,我来一一指出并解释一下。
1. 在函数 `msearch` 中,`i` 的初始值应该是字符串的长度减1,而不是0。因为字符串的最后一个字符是 `\0`,不应该在寻找字符的过程中被考虑进去。
2. 在函数 `msearch` 中,应该使用循环语句 `while` 而不是 `if`,因为需要在整个字符串中查找字符。
3. 在函数 `msearch` 中,当找到字符时,应该返回 `i` 而不是 `i` 的相反数,因为这个函数的功能是返回字符在字符串中的下标。
4. 在函数 `main` 中,应该使用 `gets` 函数来输入字符串,而不是 `putchar` 函数,因为 `putchar` 函数只能输出单个字符。
5. 在函数 `main` 中,调用 `msearch` 函数时,应该传入字符串 `s` 而不是 `s[50]`,因为 `s[50]` 表示字符串中下标为50的字符,而不是整个字符串。
6. 在函数 `main` 中,应该在输入字符串 `s` 后再输入要查找的字符 `ch`,否则程序会直接读取输入缓冲区中的一个字符。
7. 在函数 `main` 中,应该将 `s` 中的内容输出来,以便用户知道自己输入的字符串是什么。
下面是修改后的代码:
```
#include <stdio.h>
#include <string.h>
int msearch(char s[], char ch) {
int i = strlen(s) - 1;
while (i >= 0) {
if (s[i] == ch)
return i;
i--;
}
return -1;
}
void main() {
char s[50], ch;
int pot;
printf("请输入一个字符串:\n");
gets(s);
printf("你输入的字符串是:%s\n", s);
printf("请输入要查找的字符:\n");
scanf("%c", &ch);
pot = msearch(s, ch);
if (pot != -1)
printf("%c这个字符的下标是%d\n", ch, pot);
else
printf("查找不成功!\n");
}
```
相关推荐
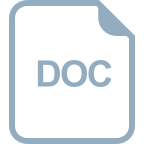
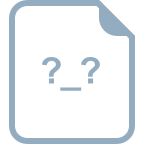
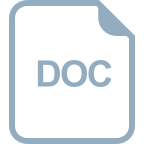















