list1.stream().forEach((p) -> { List<String> stringList = new ArrayList<>(); List<SysRole> roles = sysUserService.findRolesByUserId(p.getId()); if (!CollectionUtils.isEmpty(roles)){ for (SysRole role : roles) { stringList.add(role.getName()); } } SysDept byId = sysDeptService.getById(p.getDeptId()); p.setRoleNames(StringUtils.join(stringList,",")); p.setDeptName(byId.getName()); }); 帮我优化下这段代码
时间: 2023-05-22 16:05:30 浏览: 56
这段代码是Java代码,使用了Java 8的Stream API对列表进行操作,在每个元素上执行一个Lambda表达式。Lambda表达式内部需要对用户角色和部门进行查询,并将查询结果拼接为一个字符串,最后对用户对象的字段进行设置。
为了优化这段代码,可以考虑使用更高效的方式来查询用户角色和部门信息,例如批量查询或者缓存查询结果。同时,可以使用并行流来并发执行Lambda表达式,提高代码的执行效率。
另外,可以把“p.setRoleNames(StringUtils.join(stringList,','));”和“p.setDeptName(byId.getName());”合并到一个Lambda表达式中,避免重复遍历列表的开销。
总的来说,这段代码的优化需要根据具体的业务场景来确定,需要综合考虑执行效率和代码的可读性、可维护性等因素。
相关问题
优化代码 List<String> deptCodes = new ArrayList<>();
对于这个问题,我建议您可以使用Java 8的Stream API来优化代码,如下所示:
List<String> deptCodes = new ArrayList<>();
// 假设您已经将一些字符串添加到deptCodes中
List<String> optimizedDeptCodes = deptCodes.stream().distinct().collect(Collectors.toList());
这段代码使用了Stream API中的distinct()方法来去重,并使用collect()方法将结果收集到一个新的List中。这样可以避免使用传统的for循环或者foreach循环来进行去重操作,使代码更加简洁和易于维护。
用Java将List<List<String>>转换成List<String>
在Java中,我们可以使用forEach循环和Stream API来将List<List<String>>转换为List<String>。
首先,我们可以使用forEach循环遍历List<List<String>>中的每个元素,然后将每个元素添加到一个新的List<String>中。代码示例如下:
```java
List<List<String>> nestedList = new ArrayList<>();
nestedList.add(Arrays.asList("element1", "element2"));
nestedList.add(Arrays.asList("element3", "element4"));
List<String> flatList = new ArrayList<>();
nestedList.forEach(subList -> flatList.addAll(subList));
```
另一种方法是使用Stream API的flatMap操作,它将嵌套的列表展平为一个单独的列表。代码示例如下:
```java
List<List<String>> nestedList = new ArrayList<>();
nestedList.add(Arrays.asList("element1", "element2"));
nestedList.add(Arrays.asList("element3", "element4"));
List<String> flatList = nestedList.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
```
以上两种方法都可以将List<List<String>>转换为List<String>。请根据具体需求选择适合的方法。<span class="em">1</span><span class="em">2</span><span class="em">3</span><span class="em">4</span>
相关推荐
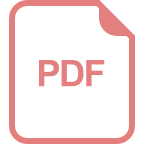
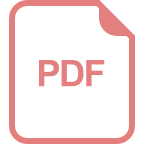
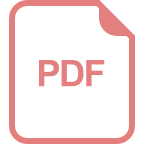












