import ord def mc(s): s=s.lower() new_str = "" for char in s: if char.isalpha(): new_char = chr((ord(char.lower()) - 97 + 3) % 26 + 97) new_str += new_char else: new_str += char return new_str input_str = input() encrypted_str = mc(input_str) print(encrypted_str) 这个代码哪里错了
时间: 2024-01-30 19:02:25 浏览: 23
这个代码的问题在于`import ord`语句不正确,应该修改为`from builtins import ord`,另外需要在第一行加入`#`符号表示注释。下面是修改后的代码:
```
# 正确的import语句
from builtins import ord
def mc(s):
s = s.lower()
new_str = ""
for char in s:
if char.isalpha():
new_char = chr((ord(char.lower()) - 97 + 3) % 26 + 97)
new_str += new_char
else:
new_str += char
return new_str
input_str = input()
encrypted_str = mc(input_str)
print(encrypted_str)
```
这样就可以正确地运行这个脚本了。
相关问题
将#!/usr/bin/env python2.7 -- coding: UTF-8 -- import time import cv2 from PIL import Image import numpy as np from PIL import Image if name == 'main': rtsp_url = "rtsp://127.0.0.1:8554/live" cap = cv2.VideoCapture(rtsp_url) #判断摄像头是否可用 #若可用,则获取视频返回值ref和每一帧返回值frame if cap.isOpened(): ref, frame = cap.read() else: ref = False #间隔帧数 imageNum = 0 sum=0 timeF = 24 while ref: ref,frame=cap.read() sum+=1 #每隔timeF获取一张图片并保存到指定目录 #"D:/photo/"根据自己的目录修改 if (sum % timeF == 0): # 格式转变,BGRtoRGB frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB) # 转变成Image frame = Image.fromarray(np.uint8(frame)) frame = np.array(frame) # RGBtoBGR满足opencv显示格式 frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR) imageNum = imageNum + 1 cv2.imwrite("/root/Pictures/Pictures" + str(imageNum) + '.png', frame) print("success to get frame") #1毫秒刷新一次 k = cv2.waitKey(1) #按q退出 #if k==27:则为按ESC退出 if k == ord('q'): cap.release() break和#!/usr/bin/env python2.7 coding=UTF-8 import os import sys import cv2 from pyzbar import pyzbar def main(image_folder_path, output_file_name): img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))] qr_codes_found = [] print("Image files:") for img_file in img_files: print(img_file) for img_file in img_files: img_path = os.path.join(image_folder_path,img_file) img = cv2.imread(img_path) barcodes = pyzbar.decode(img) for barcode in barcodes: if barcode.type == 'QRCODE': qr_data = barcode.data.decode("utf-8") qr_codes_found.append((img_file, qr_data)) unique_qr_codes = [] for file_name, qr_content in qr_codes_found: if qr_content not in unique_qr_codes: unique_qr_codes.append(qr_content) with open(output_file_name,'w') as f: for qr_content in unique_qr_codes: f.write("{}\n".format(qr_content)) if name == "main": image_folder_path = '/root/Pictures' output_file_name = 'qr_codes_found.txt' main(image_folder_path,output_file_name)合并成一个代码
以下是将两段代码合并为一个代码的结果:
```python
#!/usr/bin/env python2.7
# -*- coding: UTF-8 -*-
import time
import cv2
from PIL import Image
import numpy as np
from PIL import Image
import os
import sys
from pyzbar import pyzbar
def main(image_folder_path, output_file_name):
img_files = [f for f in os.listdir(image_folder_path) if f.endswith(('.png'))]
qr_codes_found = []
print("Image files:")
for img_file in img_files:
print(img_file)
for img_file in img_files:
img_path = os.path.join(image_folder_path,img_file)
img = cv2.imread(img_path)
barcodes = pyzbar.decode(img)
for barcode in barcodes:
if barcode.type == 'QRCODE':
qr_data = barcode.data.decode("utf-8")
qr_codes_found.append((img_file, qr_data))
unique_qr_codes = []
for file_name, qr_content in qr_codes_found:
if qr_content not in unique_qr_codes:
unique_qr_codes.append(qr_content)
with open(output_file_name,'w') as f:
for qr_content in unique_qr_codes:
f.write("{}\n".format(qr_content))
if __name__ == '__main__':
rtsp_url = "rtsp://127.0.0.1:8554/live"
cap = cv2.VideoCapture(rtsp_url)
# 判断摄像头是否可用
# 若可用,则获取视频返回值ref和每一帧返回值frame
if cap.isOpened():
ref, frame = cap.read()
else:
ref = False
# 间隔帧数
imageNum = 0
sum = 0
timeF = 24
while ref:
ref, frame = cap.read()
sum += 1
# 每隔timeF获取一张图片并保存到指定目录
# "D:/photo/"根据自己的目录修改
if (sum % timeF == 0):
# 格式转变,BGRtoRGB
frame = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
# 转变成Image
frame = Image.fromarray(np.uint8(frame))
frame = np.array(frame)
# RGBtoBGR满足opencv显示格式
frame = cv2.cvtColor(frame, cv2.COLOR_RGB2BGR)
imageNum = imageNum + 1
cv2.imwrite("/root/Pictures/Pictures" + str(imageNum) + '.png', frame)
print("success to get frame")
# 1毫秒刷新一次
k = cv2.waitKey(1)
# 按q退出
# 如果按下的是q键,则退出循环
if k == ord('q'):
cap.release()
image_folder_path = '/root/Pictures'
output_file_name = 'qr_codes_found.txt'
main(image_folder_path,output_file_name)
```
这样,代码中的摄像头捕获和图像识别逻辑将在同一个程序中运行,并且在摄像头捕获每一帧图像后会进行图像识别操作。您可以根据需要对代码进行进一步的调整和优化。
AN = input("请输入A-L之间任意一字母:") class colock: def __init__(self,hourse): self.hourse = hourse def ZM(self,hourse): str_1 = 'ABCDEFGHIJKL' s=0 for i in str_1: if i == self.hourse: if ord(i) > ord('@'): s = ord(i) - ord('@') else : s = ord('@')-ord('i') x = colock(AN) print("时针时间为:",x.ZM())代码改进
根据您提供的代码,可以进行如下改进:
1. 在输入字母之前添加一个提示信息,让用户知道需要输入什么。
2. 在ZM方法中,应该返回计算出的时间s,而不是直接打印结果。
3. 在创建colock对象时,应该将用户输入的字母作为参数传递给构造函数。
改进后的代码如下:
```
AN = input("请输入A-L之间任意一字母:")
class colock:
def __init__(self, hourse):
self.hourse = hourse
def ZM(self):
str_1 = 'ABCDEFGHIJKL'
s = 0
for i in str_1:
if i == self.hourse:
if ord(i) > ord('@'):
s = ord(i) - ord('@')
else:
s = ord('@') - ord('i')
return s
x = colock(AN)
print("时针时间为:", x.ZM())
```
注意,这里的代码只是针对您提供的代码进行了改进,如果还有其他问题,可以随时提出来哦。
相关推荐












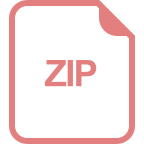