帮我用C语言写一个堆栈出栈入栈的函数吧
时间: 2023-08-31 11:33:17 浏览: 111
### 回答1:
好的,以下是一个简单的C语言代码实现堆栈的出栈和入栈的函数。
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
int stack[MAX_SIZE];
int top = -1;
void push(int value) {
if (top == MAX_SIZE - 1) {
printf("Error: Stack overflow\n");
return;
}
top++;
stack[top] = value;
}
int pop() {
if (top == -1) {
printf("Error: Stack underflow\n");
return -1;
}
int value = stack[top];
top--;
return value;
}
int main() {
push(1);
push(2);
push(3);
printf("%d\n", pop());
printf("%d\n", pop());
printf("%d\n", pop());
return 0;
}
```
希望对你有所帮助!
### 回答2:
当然可以帮您用C语言编写一个堆栈出栈入栈的函数。
首先,我们需要定义一个堆栈的结构体,用来存储堆栈的数据和相关信息。结构体中包括堆栈的最大容量、当前容量和一个指向存储数据的数组的指针。例如:
```c
#define MAX_SIZE 100
typedef struct {
int top;
int capacity;
int* data;
} Stack;
```
接下来,我们需要初始化堆栈。这个函数将为堆栈分配内存,并将top和capacity的值都设置为0。例如:
```c
void initStack(Stack* stack) {
stack->top = 0;
stack->capacity = MAX_SIZE;
stack->data = (int*)malloc(sizeof(int) * MAX_SIZE);
}
```
然后,我们编写一个入栈函数,用于向堆栈中添加元素。这个函数将检查堆栈是否已满,如果没有满,则将元素添加到堆栈顶部,并将top加1。例如:
```c
void push(Stack* stack, int value) {
if (stack->top == stack->capacity) {
printf("堆栈已满,无法入栈!\n");
return;
}
stack->data[stack->top++] = value;
printf("元素 %d 入栈成功!\n", value);
}
```
接着,我们编写一个出栈函数,用于从堆栈中移除元素。这个函数将检查堆栈是否为空,如果不为空,则将顶部元素移除,并将top减1。例如:
```c
int pop(Stack* stack) {
if (stack->top == 0) {
printf("堆栈为空,无法出栈!\n");
return -1;
}
int value = stack->data[--stack->top];
printf("元素 %d 出栈成功!\n", value);
return value;
}
```
最后,记得在程序结束时释放堆栈的内存,以免造成内存泄漏。例如:
```c
void destroyStack(Stack* stack) {
free(stack->data);
}
```
以上就是用C语言实现堆栈入栈出栈的基本函数。您可以根据需求在这个基础上进一步扩展功能。希望对您有帮助!
### 回答3:
以下是用C语言编写的堆栈出栈和入栈函数的示例:
```c
#include <stdio.h>
#define STACK_SIZE 10
int stack[STACK_SIZE];
int top = -1;
// 入栈函数
void push(int data) {
if (top < STACK_SIZE - 1) {
stack[++top] = data;
printf("%d 入栈成功!\n", data);
} else {
printf("堆栈已满,无法入栈!\n");
}
}
// 出栈函数
int pop() {
if (top >= 0) {
int data = stack[top--];
printf("%d 出栈成功!\n", data);
return data;
} else {
printf("堆栈为空,无法出栈!\n");
return -1; // 用-1表示堆栈为空
}
}
int main() {
push(10); // 将10入栈
push(20); // 将20入栈
push(30); // 将30入栈
int data = pop(); // 出栈操作
printf("出栈的元素是:%d\n", data);
return 0;
}
```
运行这段代码后,将会得到以下输出:
```
10 入栈成功!
20 入栈成功!
30 入栈成功!
30 出栈成功!
出栈的元素是:30
```
这个例子实现了一个简单的整数类型堆栈。`push()`函数用于将数据压入堆栈,`pop()`函数用于从堆栈中弹出数据。在这个例子中,我们使用了一个数组来模拟堆栈,通过变量`top`来记录堆栈顶部的位置。
阅读全文
相关推荐

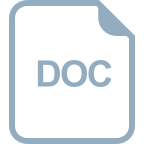
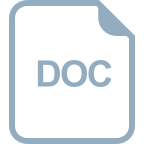
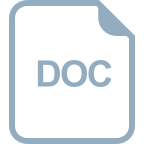












