帮我编写可以运行的并且具有以下功能的一个C语言程序:1.能 利用“数组”作为存储结构方法实现“堆栈”的基本操作,包括:创建堆栈、入栈、出栈、空栈、满栈等操作。 2.能 利用堆栈完成,将一组输入的数据逆序输出。依次入栈的数据如下:a b c d e f,依次出栈的数据如下:f e d c b a 3.能 利用“数组”作为存储结构方法实现“队列”的基本操作,包括:创建队列、入队列、出队列、队列满、队列空等基本操作。 4. 能利用“堆栈”和“队列”,对输入的一组“字符串”判断是否“回文”
时间: 2023-06-27 12:05:15 浏览: 115
以下是一个可以实现上述功能的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 10 // 堆栈和队列的最大容量
/* 堆栈结构体定义 */
typedef struct {
int top; // 栈顶指针
char data[MAX_SIZE]; // 存储数据的数组
} Stack;
/* 队列结构体定义 */
typedef struct {
int front; // 队头指针
int rear; // 队尾指针
char data[MAX_SIZE]; // 存储数据的数组
} Queue;
/* 创建空堆栈 */
void createStack(Stack *s) {
s->top = -1;
}
/* 判断堆栈是否为空 */
int isEmptyStack(Stack *s) {
return s->top == -1;
}
/* 判断堆栈是否已满 */
int isFullStack(Stack *s) {
return s->top == MAX_SIZE - 1;
}
/* 入栈 */
void push(Stack *s, char item) {
if (isFullStack(s)) {
printf("Error: stack is full\n");
exit(1);
}
s->top++;
s->data[s->top] = item;
}
/* 出栈 */
char pop(Stack *s) {
if (isEmptyStack(s)) {
printf("Error: stack is empty\n");
exit(1);
}
char item = s->data[s->top];
s->top--;
return item;
}
/* 创建空队列 */
void createQueue(Queue *q) {
q->front = q->rear = -1;
}
/* 判断队列是否为空 */
int isEmptyQueue(Queue *q) {
return q->front == -1;
}
/* 判断队列是否已满 */
int isFullQueue(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
/* 入队列 */
void enqueue(Queue *q, char item) {
if (isFullQueue(q)) {
printf("Error: queue is full\n");
exit(1);
}
if (isEmptyQueue(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_SIZE;
}
q->data[q->rear] = item;
}
/* 出队列 */
char dequeue(Queue *q) {
if (isEmptyQueue(q)) {
printf("Error: queue is empty\n");
exit(1);
}
char item = q->data[q->front];
if (q->front == q->rear) {
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_SIZE;
}
return item;
}
/* 判断字符串是否回文 */
int isPalindrome(char *str) {
Stack s;
Queue q;
int len = 0;
while (str[len] != '\0' && len < MAX_SIZE) {
len++;
}
createStack(&s);
createQueue(&q);
for (int i = 0; i < len; i++) {
push(&s, str[i]);
enqueue(&q, str[i]);
}
for (int i = 0; i < len; i++) {
if (pop(&s) != dequeue(&q)) {
return 0;
}
}
return 1;
}
/* 主函数 */
int main() {
Stack s;
Queue q;
// 测试堆栈
printf("Stack Test:\n");
createStack(&s);
push(&s, 'a');
push(&s, 'b');
push(&s, 'c');
while (!isEmptyStack(&s)) {
printf("%c ", pop(&s));
}
printf("\n\n");
// 测试队列
printf("Queue Test:\n");
createQueue(&q);
enqueue(&q, 'a');
enqueue(&q, 'b');
enqueue(&q, 'c');
while (!isEmptyQueue(&q)) {
printf("%c ", dequeue(&q));
}
printf("\n\n");
// 测试回文
char str[MAX_SIZE];
printf("Enter a string to check if it is a palindrome: ");
scanf("%s", str);
if (isPalindrome(str)) {
printf("%s is a palindrome.\n", str);
} else {
printf("%s is not a palindrome.\n", str);
}
return 0;
}
```
这个程序中,我们首先定义了堆栈和队列的结构体,并实现了它们的基本操作。然后,我们利用堆栈和队列,实现了一个判断字符串是否回文的函数,该函数先将字符串压入堆栈和队列中,然后依次从堆栈和队列中弹出字符,比较它们是否相等。最后,我们在主函数中进行了堆栈、队列和回文测试。
阅读全文
相关推荐
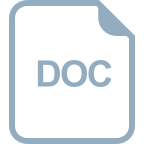
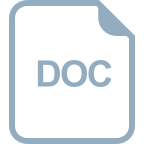
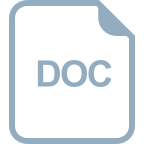







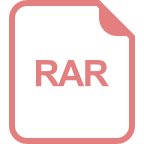
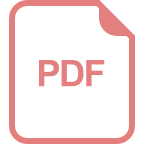
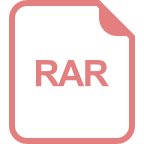
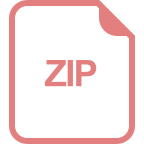
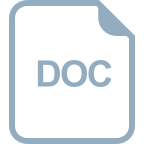
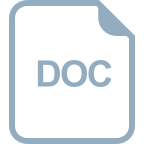


