antd vue tabs + router 实现标签栏
时间: 2023-09-11 15:07:46 浏览: 66
Ant Design Vue 中的 Tabs 组件可以与 Vue Router 配合使用,实现标签栏的功能。具体步骤如下:
1. 在路由配置中,添加 `meta` 字段用于标识当前路由是否需要在标签栏中显示,例如:
```javascript
const routes = [
{
path: '/',
name: 'Home',
component: Home,
meta: {
title: '首页',
keepAlive: true, // 是否缓存组件
showTab: true, // 是否在标签栏中显示
},
},
// ...
];
```
2. 在 App.vue 中,使用 Tabs 组件来渲染标签栏,并使用 `router-view` 组件来渲染当前路由对应的组件:
```html
<template>
<div>
<a-tabs v-model:selectedTabKey="selectedTabKey"
type="editable-card"
hide-add
@edit="handleTabEdit"
style="margin: 0 24px;">
<a-tab-pane v-for="(tab, index) in tabs"
:key="tab.key"
:tab="tab.title"
:closable="index !== 0"
@close="handleTabClose(index)">
</a-tab-pane>
</a-tabs>
<router-view />
</div>
</template>
<script>
export default {
data() {
return {
selectedTabKey: '/',
tabs: [
{
key: '/',
title: '首页',
},
],
};
},
created() {
const { path, meta } = this.$route;
if (meta.showTab) {
this.selectedTabKey = path;
this.addTab(path, meta.title);
}
},
methods: {
addTab(key, title) {
const index = this.tabs.findIndex((tab) => tab.key === key);
if (index === -1) {
this.tabs.push({
key,
title,
});
}
},
removeTab(key) {
const index = this.tabs.findIndex((tab) => tab.key === key);
if (index !== -1) {
this.tabs.splice(index, 1);
}
},
handleTabClose(index) {
const { key } = this.tabs[index];
this.removeTab(key);
this.$router.replace(this.tabs[this.tabs.length - 1].key);
},
handleTabEdit(targetKey, action) {
if (action === 'add') {
this.$router.push(targetKey);
} else if (action === 'remove') {
this.handleTabClose(this.tabs.findIndex((tab) => tab.key === targetKey));
}
},
},
};
</script>
```
在这个示例中,我们使用了 `selectedTabKey` 属性来绑定当前选中的标签页,使用 `tabs` 数组来存储所有已打开的标签页。在 `created` 钩子函数中,我们通过判断当前路由的 `meta.showTab` 字段来决定是否需要添加标签页。在 `addTab` 方法中,我们使用 `tabs` 数组来存储已打开的标签页,防止重复添加。在 `removeTab` 方法中,我们使用 `tabs` 数组来删除已关闭的标签页。在 `handleTabEdit` 方法中,我们通过判断用户的操作来决定是添加标签页还是关闭标签页。
相关推荐
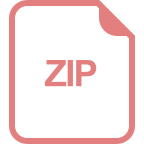
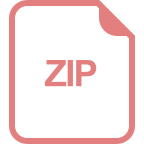
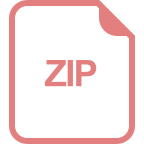














