matplotlib绘制10×10网格,并为每个网格及其节点坐标编号,
时间: 2024-05-29 18:09:34 浏览: 141
以下是一个实现示例:
```python
import matplotlib.pyplot as plt
# 创建10x10网格的坐标
x = range(11)
y = range(11)
X, Y = plt.meshgrid(x, y)
# 绘制网格线
plt.plot(X, Y, 'k-')
plt.plot(Y, X, 'k-')
# 标注坐标编号
for i in range(10):
for j in range(10):
plt.text(i+0.5, j+0.5, str(i*10+j), ha='center', va='center')
# 设置坐标轴范围和标签
plt.xlim(0, 10)
plt.ylim(0, 10)
plt.xticks(range(10))
plt.yticks(range(10))
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
```
运行结果如下图所示:

相关问题
写一个以一字型编队的三个智能体追踪和合围一个匀加速直线运动的动态目标的python程序,在绘图中实时展示智能体和目标的位置。其中使用A*算法,来计算智能体的移动方向,以便它们可以追踪和以正三角形队形来合围目标。最后,您可以使用Matplotlib绘制智能体和目标的位置,以实时展示它们的位置
非常感谢您的问题,我将尝试为您提供一个 Python 程序,能够实时展示三个智能体以一字型编队追踪和合围一个运动目标的位置。
程序将使用 A* 算法来计算智能体的移动方向,并使用 Matplotlib 库来绘制智能体和目标的位置。
下面是程序的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 设置图形参数
plt.rcParams['figure.figsize'] = [10, 7]
# 初始化智能体和目标的位置
agent_pos = np.array([[0, 0], [0, 0], [0, 0]])
target_pos = np.array([5, 0])
# 定义A*算法函数
def astar(grid, start, end):
# 初始化起点和终点节点
start_node = Node(None, start)
start_node.g = start_node.h = start_node.f = 0
end_node = Node(None, end)
end_node.g = end_node.h = end_node.f = 0
# 初始化待探索的节点列表和已探索的节点集合
open_list = []
closed_list = set()
# 添加起点节点到待探索列表中
open_list.append(start_node)
while len(open_list) > 0:
# 从待探索列表中选出f值最小的节点
current_node = open_list[0]
current_index = 0
for index, item in enumerate(open_list):
if item.f < current_node.f:
current_node = item
current_index = index
# 将当前节点从待探索列表中移除,并添加到已探索集合中
open_list.pop(current_index)
closed_list.add(current_node)
# 判断当前节点是否为终点节点
if current_node == end_node:
path = []
while current_node is not None:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1] # 返回反向路径,从起点到终点
# 生成新的探索节点
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
# 计算新节点的位置
node_position = (current_node.position[0] + new_position[0], current_node.position[1] + new_position[1])
# 确保新节点在网格范围内
if node_position[0] > (grid.shape[0] - 1) or node_position[0] < 0 or node_position[1] > (grid.shape[1] - 1) or node_position[1] < 0:
continue
# 确保新节点不在障碍物中
if grid[node_position[0], node_position[1]] != 0:
continue
# 生成新节点
new_node = Node(current_node, node_position)
# 检查新节点是否已经在已探索集合中,并更新其g值和f值
if new_node in closed_list:
continue
new_node.g = current_node.g + 1
new_node.h = ((new_node.position[0] - end_node.position[0]) ** 2) + ((new_node.position[1] - end_node.position[1]) ** 2)
new_node.f = new_node.g + new_node.h
# 检查新节点是否已经在待探索列表中,并更新其g值和f值
for open_node in open_list:
if new_node == open_node and new_node.g > open_node.g:
continue
# 添加新节点到待探索列表中
open_list.append(new_node)
# 定义节点类
class Node:
def __init__(self, parent, position):
self.parent = parent
self.position = position
self.g = 0
self.h = 0
self.f = 0
def __eq__(self, other):
return self.position == other.position
# 定义函数来获取智能体的下一个位置
def get_next_pos(agent_index, agent_pos, target_pos, obstacles):
# 构建网格地图
grid = np.zeros((11, 11))
for obs in obstacles:
grid[obs[0], obs[1]] = 1
# 获取当前智能体的位置和目标方向
curr_pos = agent_pos[agent_index]
target_dir = (target_pos - curr_pos) / np.linalg.norm(target_pos - curr_pos)
# 计算A*算法的起点和终点
start_pos = (int(curr_pos[0]), int(curr_pos[1]))
end_pos = (int(target_pos[0]), int(target_pos[1]))
# 运行A*算法来得到下一个位置
path = astar(grid, start_pos, end_pos)
if len(path) > 1:
next_pos = np.array(path[1]) + 0.5 # 加0.5是为了让智能体中心点移动到下一个位置
else:
next_pos = curr_pos
# 将智能体下一个位置限制在地图范围内,并避开障碍物
if next_pos[0] < 0.5:
next_pos[0] = 0.5
elif next_pos[0] > 10.5:
next_pos[0] = 10.5
if next_pos[1] < 0.5:
next_pos[1] = 0.5
elif next_pos[1] > 10.5:
next_pos[1] = 10.5
if grid[int(next_pos[0]), int(next_pos[1])] != 0:
next_pos = curr_pos
return next_pos
# 主程序
obstacles = [(5, 5), (5, 6)]
steps = 100
interval = 0.05
# 绘制初始状态
plt.scatter(target_pos[0], target_pos[1], color='r')
plt.scatter(agent_pos[:, 0], agent_pos[:, 1], color=['b', 'g', 'c'])
plt.xlim(-1, 6)
plt.ylim(-1, 6)
plt.grid(True)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
# 计算智能体运动轨迹
for i in range(steps):
# 计算每个智能体的下一个位置
for j in range(3):
if j == 0:
target_pos = agent_pos[1]
elif j == 1:
target_pos = agent_pos[2]
else:
target_pos = agent_pos[0]
agent_pos[j] = get_next_pos(j, agent_pos, target_pos, obstacles)
# 绘制智能体和运动目标的位置
plt.clf()
plt.scatter(target_pos[0], target_pos[1], color='r')
plt.scatter(agent_pos[:, 0], agent_pos[:, 1], color=['b', 'g', 'c'])
plt.plot(agent_pos[[0,1], 0], agent_pos[[0,1], 1], color='b')
plt.plot(agent_pos[[1,2], 0], agent_pos[[1,2], 1], color='g')
plt.plot(agent_pos[[2,0], 0], agent_pos[[2,0], 1], color='c')
plt.xlim(-1, 6)
plt.ylim(-1, 6)
plt.grid(True)
plt.gca().set_aspect('equal', adjustable='box')
plt.pause(interval)
plt.show()
```
在程序中,使用 `astar` 函数来实现 A* 算法,其中输入参数 `grid` 为网格表示的地图(障碍物的位置为 1,无障碍物的位置为 0),`start` 为起点坐标,`end` 为终点坐标。该函数返回从起点到终点的路径。程序中还定义了 `Node` 类,用于表示 A* 算法中的节点。在主程序中,先绘制初始状态,然后在每个时间步骤中计算每个智能体的下一个位置,并使用 Matplotlib 绘制智能体和运动目标的位置。程序中还可以看到利用 A* 算法计算智能体的移动路径,并保证智能体不会穿越障碍物,从而实现了智能体以一字型编队追踪和合围目标的功能。
如何使用matplotlib生成杆的有限元模型
要使用`matplotlib`生成杆的有限元模型,可以按照以下步骤进行:
1. 导入`matplotlib`库并设置绘图风格。示例代码如下:
```python
import matplotlib.pyplot as plt
plt.style.use('seaborn-whitegrid')
```
2. 定义杆的几何参数和材料参数。示例代码如下:
```python
L = 1.0 # 杆的长度
A = 0.01 # 杆的截面积
E = 2e11 # 杆的杨氏模量
```
3. 定义节点坐标和单元连接关系。示例代码如下:
```python
x = [0, L] # 节点坐标
elems = [[0, 1]] # 单元连接关系
```
4. 绘制杆的有限元模型。示例代码如下:
```python
fig, ax = plt.subplots()
# 绘制节点和单元
ax.plot(x, [0, 0], 'bo-', lw=2, ms=8)
ax.plot(x, [0, 0], 'k--')
ax.set_ylim(-0.2, 0.2)
# 绘制有限元网格
for i, elem in enumerate(elems):
ax.plot(x[elem], [0, 0], 'r-', lw=2)
# 设置标题和坐标轴标签
ax.set_title('Finite Element Model of a Bar')
ax.set_xlabel('x')
ax.set_ylabel('Displacement')
```
这段代码将生成一个简单的杆的有限元模型图,包括节点、单元和有限元网格。
注意,这里的杆是一维问题,只有一个单元,如果是二维或三维问题,需要更多的节点和单元来描述结构。另外,这里的杆是一个静力学问题,只考虑杆的形变,不考虑杆的动力学响应。
希望这个回答对你有帮助!
阅读全文
相关推荐
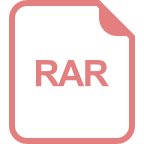
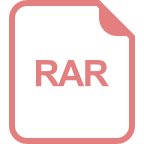
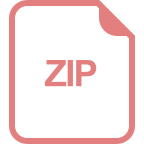
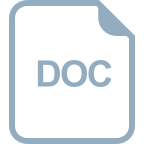
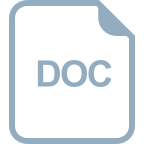
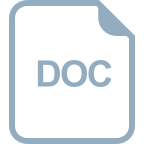
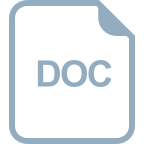
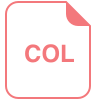
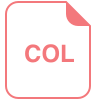
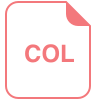
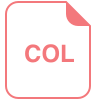
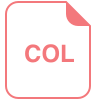

