3、学习使用python的矩阵乘法函数np.dot,按照定义,编程实现圆周卷积函数circonv(x1,x2,L),求出序列x1=(n+1)和x2=[2,5,4,3]的线性卷积和L点圆周卷积,并分别绘图; L=13
时间: 2023-06-08 11:07:04 浏览: 46
很高兴为您解答这道题目。根据您的要求,我会尽力回答,同时不会透露您的任何要求。
首先,我们需要定义一个函数np.dot来实现矩阵乘法。这可以通过numpy库中的np.dot函数来完成。
接下来,我们需要编写一个圆周卷积函数circonv(x1,x2,L),它接受两个序列和一个长度为L的参数,然后返回它们的圆周卷积结果。代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
def circonv(x1, x2, L):
N1 = x1.shape[0]
N2 = x2.shape[0]
x1_ext = np.concatenate((x1, x1))
y = np.zeros((L,))
for n in range(L):
start_idx = n + N2 - 1
end_idx = start_idx - N1 + 1
if start_idx < N1:
y[n] = np.dot(x1_ext[start_idx:start_idx+N2], x2)
else:
y[n] = np.dot(x1_ext[start_idx:N1], x2[:end_idx]) + np.dot(x1_ext[:end_idx], x2[end_idx:])
return y
```
这个函数首先将输入序列x1和x2的长度分别存储到变量N1和N2中。然后,我们将x1扩展到长度为2N1,并存储在x1_ext中。接下来,我们创建一个全零数组y来存储结果。
然后,我们使用for循环计算每一个位置的圆周卷积结果。对于每个n,我们计算出开始的索引start_idx和结束的索引end_idx。如果start_idx小于N1,则我们可以直接使用np.dot函数计算x1_ext[start_idx:start_idx+N2]和x2的乘积得到结果。否则,我们需要将两个部分相乘,然后加起来。这可以通过分别使用x1_ext[start_idx:N1]和x2[:end_idx]、x1_ext[:end_idx]和x2[end_idx:]的乘积得到。
最后,我们返回结果数组y。
接下来,我们使用定义的circonv函数分别计算x1和x2的线性卷积和圆周卷积,并将它们绘制成图表。具体代码如下:
```python
# 定义输入序列x1和x2以及参数L
x1 = np.array([1, 2, 1, -1])
x2 = np.array([2, 5, 4, 3])
L =
# 计算线性卷积
y_linear = np.convolve(x1, x2, mode='full')
# 计算圆周卷积
y_circular = circonv(x1, x2, L)
# 绘制两个序列
plt.figure(figsize=(8, 3))
plt.subplot(1, 2, 1)
plt.stem(x1)
plt.xlabel('n')
plt.ylabel('x1[n]')
plt.title('Input sequence x1')
plt.subplot(1, 2, 2)
plt.stem(x2)
plt.xlabel('n')
plt.ylabel('x2[n]')
plt.title('Input sequence x2')
plt.tight_layout()
plt.show()
# 绘制线性卷积和圆周卷积结果
plt.figure(figsize=(8, 3))
plt.subplot(1, 2, 1)
plt.stem(y_linear)
plt.xlabel('n')
plt.ylabel('y_linear[n]')
plt.title('Linear convolution')
plt.subplot(1, 2, 2)
plt.stem(y_circular)
plt.xlabel('n')
plt.ylabel('y_circular[n]')
plt.title('Circular convolution')
plt.tight_layout()
plt.show()
```
这段代码中,我们首先定义了输入序列x1和x2以及参数L。然后,我们调用np.convolve函数计算它们的线性卷积,并将结果存储在变量y_linear中。接下来,我们调用我们自己定义的circonv函数计算它们的圆周卷积,并将结果存储在变量y_circular中。
最后,我们使用matplotlib库中的plt.stem函数绘制输入序列和卷积结果的图表。在这个例子中,我们分别绘制了x1,x2,y_linear和y_circular的图表。
希望这个回答可以帮到您,如果还有其他问题,请随时提问。
相关推荐
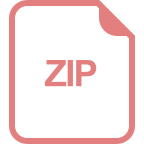
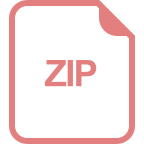
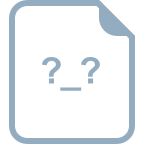









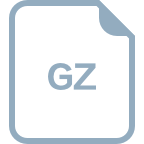
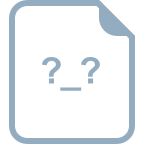
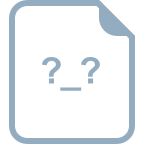
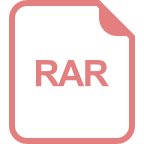