用java写一个从字符串中找出邮箱地址,并进行脱敏处理的算法
时间: 2024-03-22 16:41:51 浏览: 204
可以使用正则表达式来找出字符串中的邮箱地址,然后将其中的用户名部分使用 * 号来进行脱敏处理。
下面是一个简单的 Java 代码示例:
```java
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class EmailMasking {
public static void main(String[] args) {
String input = "Please send the email to john.doe@example.com or jane.doe@example.com";
String patternString = "\\b([a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,})\\b";
Pattern pattern = Pattern.compile(patternString);
Matcher matcher = pattern.matcher(input);
StringBuffer sb = new StringBuffer();
while (matcher.find()) {
String email = matcher.group(1);
String masked = maskEmail(email);
matcher.appendReplacement(sb, masked);
}
matcher.appendTail(sb);
System.out.println(sb.toString());
}
private static String maskEmail(String email) {
int index = email.indexOf("@");
String username = email.substring(0, index);
String domain = email.substring(index);
int length = username.length();
if (length <= 2) {
return "***" + domain;
} else {
int maskLength = length / 2;
String prefix = username.substring(0, length - maskLength);
String suffix = username.substring(length - maskLength);
String mask = "*".repeat(maskLength);
return prefix + mask + suffix + domain;
}
}
}
```
输出结果为:
```
Please send the email to j**n.doe@example.com or j**e.doe@example.com
```
该算法使用正则表达式来匹配字符串中的邮箱地址,并使用 StringBuffer 来进行替换。其中 maskEmail 方法用于对邮箱地址进行脱敏处理,如果用户名部分长度小于等于 2,则使用三个星号进行脱敏,否则使用一半的星号进行脱敏。
阅读全文
相关推荐
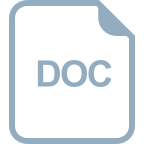
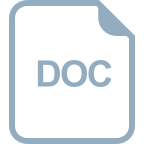
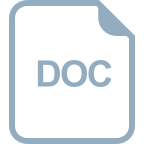
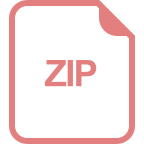
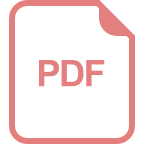
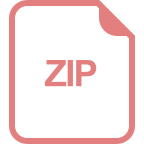
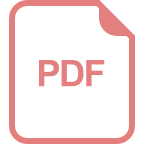
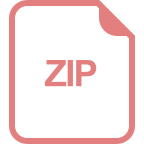
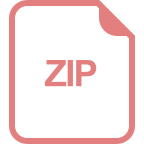
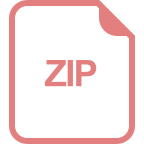
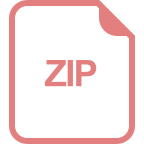
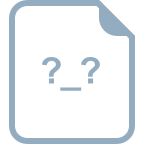
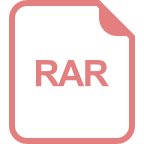
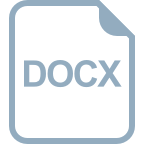