python实现生产者消费者问题
时间: 2023-12-04 10:57:44 浏览: 33
生产者消费者问题是指有一定数量的生产者和消费者,共同使用一个共享资源,生产者往该共享资源中添加数据,而消费者从该共享资源中取出数据,两者之间需要协调合作,保证数据的生产和消费的有效性。
以下是一个简单的 Python 实现代码:
```python
import threading
import time
# 定义生产者类
class ProducerThread(threading.Thread):
def __init__(self, name, queue):
threading.Thread.__init__(self, name=name)
self.queue = queue
def run(self):
while True:
if self.queue.qsize() < 10: # 设置缓冲区最大值
self.queue.put(1)
print(f'{self.name} producing one element, queue size is {self.queue.qsize()}')
time.sleep(1)
else:
time.sleep(1)
# 定义消费者类
class ConsumerThread(threading.Thread):
def __init__(self, name, queue):
threading.Thread.__init__(self, name=name)
self.queue = queue
def run(self):
while True:
if self.queue.qsize() > 0:
self.queue.get()
print(f'{self.name} consuming one element, queue size is {self.queue.qsize()}')
time.sleep(1)
else:
time.sleep(1)
if __name__ == '__main__':
q = Queue(10) # 设置缓冲区大小
p1 = ProducerThread('producer1', q)
p2 = ProducerThread('producer2', q)
c1 = ConsumerThread('consumer1', q)
c2 = ConsumerThread('consumer2', q)
p1.start()
p2.start()
c1.start()
c2.start()
```
其中,使用了 Python 自带的队列 Queue 模块来实现缓冲区,并采用了多线程的方式对生产者和消费者进行协作,保证不会出现同时对缓冲区进行操作的情况。在主函数中,创建了四个线程,分别是两个生产者和两个消费者,并启动它们。每个线程执行的过程中,都会不断地进行生产和消费,中间加入了一段等待时间,从而使得整个执行过程具有一定的可控性。
相关推荐
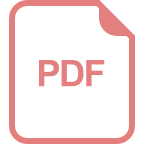
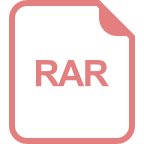














