JPanel formPanel = new JPanel(); formPanel.setLayout(new GridLayout(2, 2)); usernameLabel = new JLabel("Username:"); usernameField = new JTextField(); passwordLabel = new JLabel("Password:"); passwordField = new JPasswordField(); formPanel.add(usernameLabel); formPanel.add(usernameField); formPanel.add(passwordLabel); formPanel.add(passwordField); add(formPanel, BorderLayout.CENTER);怎么使其中文本框适应大小,居中对齐
时间: 2023-12-27 08:05:34 浏览: 72
你可以使用JTextField的setHorizontalAlignment方法将文本框中的文本居中对齐,通过设置preferredSize属性来适应大小。
例如:
```
usernameField.setPreferredSize(new Dimension(200, 30)); // 设置文本框大小
usernameField.setHorizontalAlignment(JTextField.CENTER); // 设置文本居中对齐
```
同样的,也可以用同样的方式对passwordField进行设置。
阅读全文
相关推荐
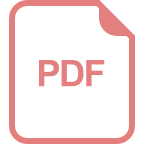
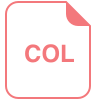
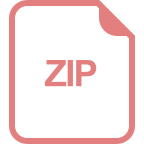
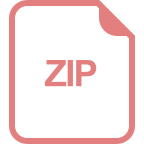
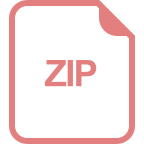
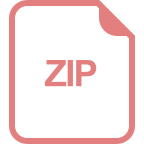
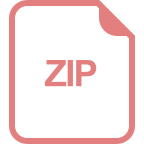
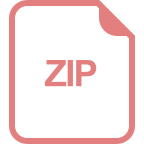
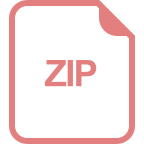
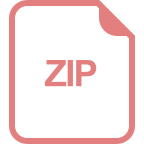
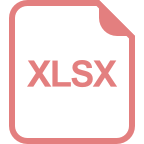
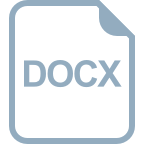
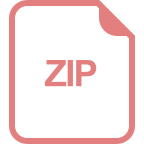
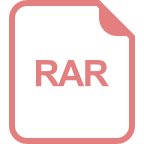
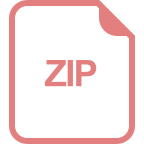
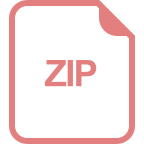