vue2使用高德地图如何初始化加载显示各个省份
时间: 2023-12-15 10:05:14 浏览: 269
要在Vue2中使用高德地图并显示各个省份,您需要遵循以下步骤:
1. 在项目中安装高德地图JavaScript API。
2. 在Vue组件中引入高德地图JavaScript API。
3. 在Vue组件中使用高德地图JavaScript API初始化地图。
4. 使用高德地图JavaScript API加载各个省份的边界数据并在地图上显示。
下面是一个示例代码,演示如何在Vue2中使用高德地图来显示各个省份:
```
<template>
<div id="map-container"></div>
</template>
<script>
import AMapLoader from '@amap/amap-jsapi-loader';
export default {
name: 'Map',
data() {
return {
map: null,
};
},
async mounted() {
await AMapLoader.load({
key: 'your_amap_key',
version: '2.0',
plugins: ['AMap.Geocoder'],
});
const map = new AMap.Map('map-container', {
zoom: 4,
center: [116.397428, 39.90923],
});
this.map = map;
const geoJSON = await this.getBoundaryData();
map.on('complete', () => {
map.setFitView();
});
map.setFeatures(['bg', 'road']);
map.setMapStyle('amap://styles/whitesmoke');
map.plugin('AMap.CustomLayer', () => {
const canvas = document.createElement('canvas');
canvas.width = map.getSize().width;
canvas.height = map.getSize().height;
const customLayer = new AMap.CustomLayer(canvas, {
zooms: [1, 20],
zIndex: 100,
});
customLayer.render = () => {
if (!geoJSON) {
return;
}
const ctx = canvas.getContext('2d');
const zoom = map.getZoom();
ctx.clearRect(0, 0, canvas.width, canvas.height);
geoJSON.features.forEach((feature) => {
const provinceName = feature.properties.name;
const isHovered = provinceName === this.hoveredProvinceName;
const isSelected = provinceName === this.selectedProvinceName;
const isHighlighted = isHovered || isSelected;
if (isHighlighted) {
ctx.lineWidth = 3;
ctx.strokeStyle = 'rgba(255, 255, 255, 0.7)';
ctx.fillStyle = 'rgba(255, 255, 255, 0.5)';
} else {
ctx.lineWidth = 1;
ctx.strokeStyle = 'rgba(0, 0, 0, 0.2)';
ctx.fillStyle = 'rgba(0, 0, 0, 0.1)';
}
ctx.beginPath();
feature.geometry.coordinates.forEach((polygon) => {
polygon.forEach((ring) => {
ring.forEach((point, index) => {
const [x, y] = map.lngLatToContainer([point[0], point[1]]);
if (index === 0) {
ctx.moveTo(x, y);
} else {
ctx.lineTo(x, y);
}
});
});
});
ctx.closePath();
ctx.stroke();
ctx.fill();
});
};
customLayer.setMap(map);
});
},
methods: {
async getBoundaryData() {
const response = await fetch('/path/to/your/province/boundary/data');
const data = await response.json();
return data;
},
},
};
</script>
<style>
#map-container {
width: 100%;
height: 100%;
}
</style>
```
在这个示例代码中,我们使用了高德地图JavaScript API和Vue2,来初始化地图并显示各个省份的边界。使用`AMapLoader`来加载高德地图JavaScript API,然后使用`AMap.Map`来初始化地图。在`mounted`钩子函数中,我们使用`AMap.CustomLayer`来渲染各个省份的边界。`getBoundaryData`方法中,我们加载各个省份的边界数据。
阅读全文
相关推荐
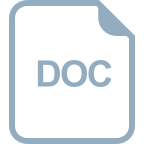
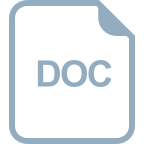
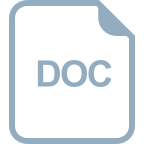
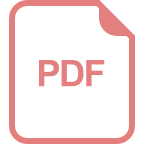
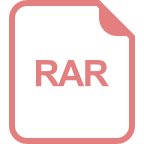


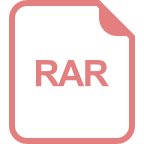
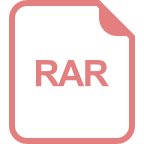
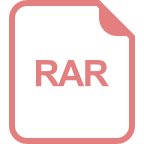
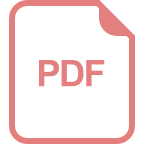
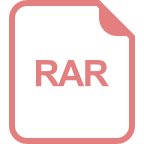
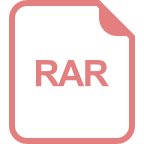
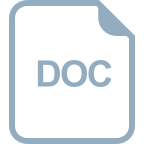



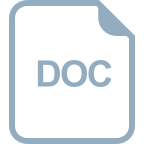
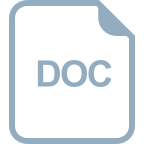