pandas去除xlsx文件重复内容
时间: 2023-08-02 18:08:46 浏览: 82
要去除Excel文件中的重复内容,可以使用pandas库中的drop_duplicates()方法。以下是一个示例代码:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('example.xlsx')
# 去除重复内容
df.drop_duplicates(inplace=True)
# 将去除重复内容后的结果写入Excel文件
df.to_excel('example.xlsx', index=False)
```
在这个示例代码中,我们首先使用`pd.read_excel()`方法读取Excel文件的内容,然后使用`drop_duplicates()`方法去除重复内容,并将结果保存在原始DataFrame中。最后,我们使用`to_excel()`方法将去除重复内容后的结果写入Excel文件中。请注意,在`to_excel()`方法中,我们将`index`参数设置为`False`,以避免将行索引写入Excel文件中。
相关问题
pandas去除xlsx文件重复内容并输出文件结果
你可以使用Pandas的`drop_duplicates`函数去除Excel文件中的重复数据,并使用`to_excel`方法输出结果到新的Excel文件中。以下是一个简单的示例代码:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('your_file.xlsx', sheet_name='your_sheet')
# 去除重复数据
df.drop_duplicates(inplace=True)
# 输出结果到新的Excel文件
df.to_excel('output_file.xlsx', sheet_name='output_sheet', index=False)
```
在上面的代码中,我们首先使用`pd.read_excel`函数从Excel文件中读取数据,然后使用`drop_duplicates`函数去除重复数据。注意,`inplace=True`表示直接对原始数据进行修改。最后,我们使用`df.to_excel`方法将去重后的结果输出到新的Excel文件中。
pandas给xlsx文件去重
要使用pandas给xlsx文件去重,可以使用drop_duplicates()方法。该方法可以基于指定的列或所有列进行去重。
以下是一个简单的示例代码:
```python
import pandas as pd
# 读取xlsx文件
df = pd.read_excel('your_file.xlsx')
# 去除重复行
df.drop_duplicates(inplace=True)
# 将结果保存到新的xlsx文件
df.to_excel('new_file.xlsx', index=False)
```
在上面的代码中,我们首先使用read_excel()方法读取xlsx文件并存储在一个DataFrame中。然后,我们使用drop_duplicates()方法来去除DataFrame中的重复行。最后,我们使用to_excel()方法将结果保存到一个新的xlsx文件中。
注意,我们将inplace参数设置为True,这将修改原始DataFrame而不是创建一个新的DataFrame。如果你想保留原始DataFrame不变,可以将inplace参数设置为False或者不指定该参数。
阅读全文
相关推荐
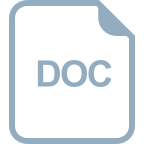
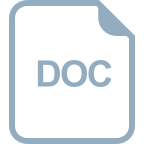
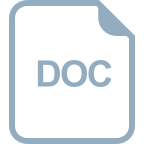
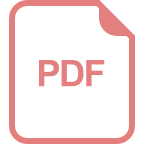
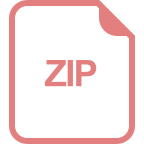
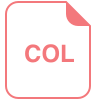
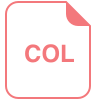










