C#连接mysql,使用窗体控件输入信息,并添加到mysql中
时间: 2024-02-27 07:54:03 浏览: 84
要在 C# 中使用窗体控件输入信息,并将其添加到 MySQL 数据库中,可以在窗体中添加相应的控件,如 `TextBox`、`Button` 等,并在按钮的点击事件中编写代码。
以下是一个简单的代码示例,假设窗体上有两个文本框控件 `txtName` 和 `txtAge`,以及一个按钮控件 `btnAdd`:
```csharp
using System;
using MySql.Data.MySqlClient;
class MyForm : Form
{
private TextBox txtName;
private TextBox txtAge;
private Button btnAdd;
public MyForm()
{
InitializeComponent();
}
private void InitializeComponent()
{
// 初始化控件
this.txtName = new TextBox();
this.txtAge = new TextBox();
this.btnAdd = new Button();
// 设置控件属性
this.txtName.Location = new Point(10, 10);
this.txtName.Size = new Size(200, 20);
this.txtAge.Location = new Point(10, 40);
this.txtAge.Size = new Size(200, 20);
this.btnAdd.Location = new Point(10, 70);
this.btnAdd.Size = new Size(100, 30);
this.btnAdd.Text = "添加";
this.btnAdd.Click += new EventHandler(btnAdd_Click);
// 添加控件到窗体
this.Controls.Add(this.txtName);
this.Controls.Add(this.txtAge);
this.Controls.Add(this.btnAdd);
}
private void btnAdd_Click(object sender, EventArgs e)
{
string connectionString = "server=localhost;user=root;password=123456;database=mydatabase";
MySqlConnection connection = new MySqlConnection(connectionString);
try
{
connection.Open();
string name = txtName.Text;
int age = int.Parse(txtAge.Text);
string sql = string.Format("INSERT INTO users (name, age) VALUES ('{0}', {1})", name, age);
MySqlCommand command = new MySqlCommand(sql, connection);
int rows = command.ExecuteNonQuery();
MessageBox.Show("已成功插入 " + rows + " 行数据。");
}
catch (Exception ex)
{
MessageBox.Show("数据插入失败:" + ex.Message);
}
finally
{
connection.Close();
}
}
}
```
在上面的示例中,我们在 `btnAdd_Click` 方法中获取文本框中的输入值,并执行 SQL INSERT 语句将其添加到 MySQL 数据库中。在执行 INSERT 语句之前,我们需要对输入值进行验证和处理,例如检查输入是否为空、是否为数字等。
请根据实际情况修改连接字符串中的参数值,并根据需要对输入值进行验证和处理。
阅读全文
相关推荐
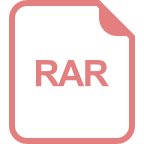
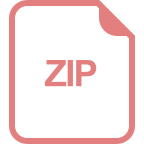
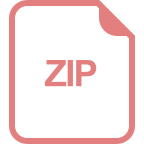















