C# 窗体应用编写一个新闻管理程序连接数据库
时间: 2023-06-20 14:10:10 浏览: 58
好的,下面是一个简单的 C# 窗体应用程序,用于连接数据库并管理新闻。
首先,您需要在 Visual Studio 中创建一个新的 C# 窗体应用程序项目。接下来,您需要添加一个 DataGridView 控件和一些 TextBox 和 Button 控件。
然后,您需要安装 MySQL 数据库,并使用 Visual Studio 的 NuGet 包管理器安装 MySQL.Data 和 MySQL.Data.Entity 包。安装完成后,您需要连接到数据库。您可以在 App.config 文件中添加以下连接字符串:
```xml
<connectionStrings>
<add name="NewsDBContext" connectionString="server=localhost;user id=root;password=yourpassword;database=newsdb"/>
</connectionStrings>
```
接下来,您需要创建一个 News 类来表示新闻,并在 NewsDBContext 类中创建一个 DbSet<News> 属性。代码如下所示:
```csharp
using System.ComponentModel.DataAnnotations;
public class News
{
[Key]
public int Id { get; set; }
[Required]
public string Title { get; set; }
[Required]
public string Content { get; set; }
}
using System.Data.Entity;
public class NewsDBContext : DbContext
{
public DbSet<News> News { get; set; }
}
```
现在,您可以在窗体应用程序中添加以下代码,以实现新闻管理功能:
```csharp
using System.Linq;
public partial class MainForm : Form
{
private NewsDBContext dbContext;
public MainForm()
{
InitializeComponent();
dbContext = new NewsDBContext();
}
private void MainForm_Load(object sender, EventArgs e)
{
RefreshDataGridView();
}
private void btnAdd_Click(object sender, EventArgs e)
{
var news = new News
{
Title = txtTitle.Text,
Content = txtContent.Text
};
dbContext.News.Add(news);
dbContext.SaveChanges();
RefreshDataGridView();
}
private void btnUpdate_Click(object sender, EventArgs e)
{
int id = int.Parse(txtId.Text);
var news = dbContext.News.FirstOrDefault(n => n.Id == id);
if (news != null)
{
news.Title = txtTitle.Text;
news.Content = txtContent.Text;
dbContext.SaveChanges();
RefreshDataGridView();
}
}
private void btnDelete_Click(object sender, EventArgs e)
{
int id = int.Parse(txtId.Text);
var news = dbContext.News.FirstOrDefault(n => n.Id == id);
if (news != null)
{
dbContext.News.Remove(news);
dbContext.SaveChanges();
RefreshDataGridView();
}
}
private void RefreshDataGridView()
{
dgvNews.DataSource = dbContext.News.ToList();
}
}
```
在上述代码中,我们首先在 MainForm 类的构造函数中创建了 NewsDBContext 实例。然后,在窗体加载事件中,我们调用 RefreshDataGridView() 方法来显示所有新闻。
接下来,我们为 btnAdd、btnUpdate 和 btnDelete 按钮添加了 Click 事件处理程序。当用户单击这些按钮时,我们将读取用户在 TextBox 控件中输入的值,并将其存储到数据库中。
最后,我们定义了一个 RefreshDataGridView() 方法,用于更新 DataGridView 控件中显示的新闻列表。该方法首先从数据库中读取所有新闻,并将其绑定到 DataGridView 控件。
现在,您可以运行该应用程序并尝试添加、更新和删除新闻。
相关推荐
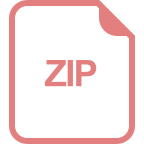
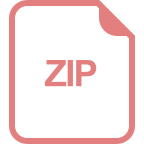
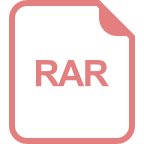














