用动态联编机制处理学生信息: 1.定义抽象类process:只提供一个纯虚函数print(); 2.定义person类,公有继承process类,定义print()成员函数显示相关信息(用前面已用过的类); 3.定义student类,公有继承person类,定义print()成员函数显示相关信息(用前面已用过的类); 4.在main()函数中,定义process指针变量调用person 对象及student对象。 说明:可将process类的定义及person类的定义放在同一个头文件中;程序存为exp_507.cpp中。
时间: 2023-07-10 13:29:33 浏览: 45
以下是实现了动态联编机制处理学生信息的代码:
```c++
#include <iostream>
#include <string>
using namespace std;
class process {
public:
virtual void print() = 0;
};
class person : public process {
protected:
string name;
int age;
char gender;
public:
person(string n, int a, char g): name(n), age(a), gender(g) {}
void print() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
}
};
class student : public person {
private:
string major;
double gpa;
public:
student(string n, int a, char g, string m, double gpa): person(n, a, g), major(m), gpa(gpa) {}
void print() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
cout << "Major: " << major << endl;
cout << "GPA: " << gpa << endl;
}
};
int main() {
process *p = nullptr;
person p1("Tom", 20, 'M');
student s1("Mary", 19, 'F', "Computer Science", 3.8);
p = &p1;
p->print();
cout << "========================" << endl;
p = &s1;
p->print();
return 0;
}
```
程序中,定义了抽象类`process`,其中只提供了一个纯虚函数`print()`。然后定义了`person`类,公有继承自`process`类,并实现`print()`函数,用于显示人员的基本信息。在`student`类中,公有继承自`person`类,同时增加了`major`和`gpa`属性,并实现了`print()`函数,用于显示学生的详细信息。在`main()`函数中,定义了`process`指针变量,并分别指向了`person`对象和`student`对象,并调用了它们的`print()`函数。由于使用了动态联编机制,所以程序能够正确调用`person`和`student`对象的`print()`函数,实现了多态性。
相关推荐
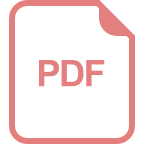
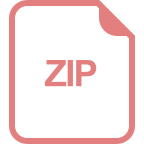















