void copy(char *m) { char n[20]; strcpy(m,n); printf("%s",n); }这串代码存在什么错误导致无法将复制的代码输出
时间: 2024-02-15 20:01:53 浏览: 33
这段代码有两个问题。
首先,`n` 数组没有被初始化,因此其内容是未定义的,拷贝到 `m` 后可能会输出无意义的字符。
其次,`n` 数组是在函数内部定义的局部变量,函数结束后,它所占用的内存空间将被释放,因此 `m` 所指向的内容也将是未定义的。
如果要解决这个问题,需要分配一个动态内存来存储拷贝的字符串,例如使用 `malloc()` 函数。同时,需要在使用完后手动释放内存,否则会出现内存泄漏的问题。以下是一种可能的修改方法:
```
void copy(char *m) {
char *n = malloc(20); // 分配一块动态内存
strcpy(n, m); // 拷贝字符串
printf("%s", n);
free(n); // 释放动态内存
}
```
相关问题
use C program Write a function to copy all the characters in the input string t starting from the m-th character to the string s.call this function in main. void strmcpy( char *t, int m, char *s ); The strmcpy function copies all characters starting from the m-th character in the input string char * t to the string char * s. If m exceeds the length of the input string, the resulting string should be an empty string.
Sure! Here's an example implementation of the `strmcpy` function in C:
```c
#include <stdio.h>
#include <string.h>
void strmcpy(char *t, int m, char *s) {
int len = strlen(t);
if (m > len) {
// m is greater than the length of t
s[0] = '\0'; // empty string
} else {
// copy characters from t to s
strcpy(s, t + m - 1);
}
}
int main() {
char t[] = "hello world";
char s[20];
int m = 3;
strmcpy(t, m, s);
printf("%s\n", s);
return 0;
}
```
In this implementation, we first find the length of the input string `t` using the `strlen` function. If `m` is greater than the length of `t`, we set `s` to an empty string by setting the first character to `\0`. Otherwise, we use the `strcpy` function to copy all characters from `t` starting from the `m`-th character to `s`. Note that we subtract 1 from `m` because string indices in C start from 0.
In the `main` function, we test the `strmcpy` function by calling it with the input string `t`, the starting index `m`, and an output string `s`. We then print the resulting string `s` to the console.
void do_check_history(user_t user, char name_copy[32], sqlite3* sqlite_p, int event_fd) { char content[128] = { 0 }; char* errmsg = NULL; // 接收错误信息指针 char** result = NULL; // 结果集指针 int row = 0; // 数据表行 int column = 0; // 数据表列 printf("开始查询[%s]的历史记录\n", name_copy); // 查找历史记录,获取结果集 char sql[1024] = { 0 }; sprintf(sql, "select * from %s ", name_copy); int ret = sqlite3_get_table(sqlite_p, sql, &result, &row, &column, &errmsg); if (ret != SQLITE_OK) { printf("历史记录数据表提取信息失败\n"); } printf("历史记录数据表提取信息成功\n"); // 向客户端回显历史记录 int index = column; // 数组下标走到第一行第一个内容 for (int i = 0; i < row; i++) { sprintf(content, "时间[%s] 单词[%s]", result[index], result[index + 1]); if ((send(event_fd, content, sizeof(content), 0)) == -1) printf("send error"); // 发送查询后的结果 // memset(content, 0, sizeof(content)); index += column; } strcpy(content, "**OVER**"); send(event_fd, content, sizeof(content), 0); // 释放错误指针空间 sqlite3_free(errmsg); // 释放结果集 sqlite3_free_table(result); }这个函数有什么不足,分析一下
这个函数存在以下不足:
1.函数没有进行输入参数的有效性校验,例如name_copy指针是否为空等,容易导致程序崩溃或者出现其他异常情况。
2.函数中使用了sprintf函数,容易造成缓冲区溢出漏洞,建议使用更安全的函数,例如snprintf。
3.函数中没有进行数据库操作的错误处理,不能及时发现和处理数据库操作的错误。
4.函数中的发送操作没有进行错误处理,如果发送失败,程序会直接退出,建议添加错误处理。
5.函数中的内容格式没有进行规范化处理,容易造成数据的混乱,建议进行格式化输出。
阅读全文
相关推荐
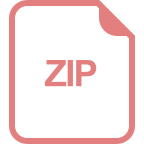
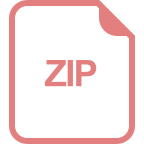
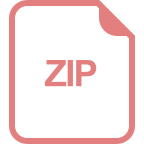














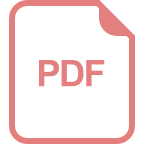