如何在Jupyter Notebook中导入数据集?
时间: 2024-02-13 21:04:19 浏览: 138
在 Jupyter Notebook 中导入数据集可以使用 pandas 库中的 read_csv() 函数,该函数可以读取 CSV 文件并将其存储为 DataFrame 对象。以下是一个简单的示例代码:
```python
import pandas as pd
# 读取 CSV 文件
df = pd.read_csv('path/to/your/dataset.csv')
# 打印前 5 行数据
print(df.head())
```
在代码中,`'path/to/your/dataset.csv'` 应替换为你实际的数据集路径。如果你的数据集不是 CSV 文件,可以使用 pandas 库中的其他读取函数,例如 read_excel()、read_json() 等。
相关问题
可以使用jupyter notebook处理数据集吗?怎么将数据集导入到jupyter notebook中并加以处理
可以使用jupyter notebook处理数据集。将数据集导入到jupyter notebook中,可以使用pandas库中的read_csv()函数读取csv格式的数据集,或者使用其他适合数据集格式的函数进行读取。读取后,可以使用pandas库中的各种函数对数据集进行处理,例如数据清洗、数据分析、数据可视化等。
jupyter notebook怎么导入数据集
在Jupyter Notebook中导入数据集可以通过以下几种方式实现:
1. 使用pandas库导入数据集:首先,确保你已经安装了pandas库。然后,在Jupyter Notebook中创建一个新的代码单元格,使用以下代码导入数据集:
```python
import pandas as pd
# 读取CSV文件
data = pd.read_csv('path_to_your_dataset.csv')
# 显示数据集的前几行
data.head()
```
在上述代码中,将`path_to_your_dataset.csv`替换为你数据集的实际路径。这将使用pandas库的`read_csv()`函数读取CSV文件,并将数据存储在名为`data`的DataFrame对象中。你可以使用`head()`函数显示数据集的前几行。
2. 使用numpy库导入数据集:如果你的数据集是以文本文件或其他格式存储的,你可以使用numpy库来导入数据。在Jupyter Notebook中创建一个新的代码单元格,使用以下代码导入数据集:
```python
import numpy as np
# 从文本文件中加载数据集
data = np.loadtxt('path_to_your_dataset.txt')
# 显示数据集的形状
print(data.shape)
```
在上述代码中将`path_to_your_dataset.txt`替换为你数据集实际路径。这将使用numpy库的`loadtxt()`函数从文本文件中加载数据,并将数据存储在名为`data`的numpy数组中。你可以使用`shape`属性来查看数据集的形状。
3. 使用其他相关库导入数据集:除了pandas和numpy,还有其他一些库可以用于导入数据集,如scikit-learn、tensorflow等。具体的导入方法取决于你使用的数据集和库。你可以查阅相关库的文档或搜索相关教程以获取更多详细信息。
阅读全文
相关推荐
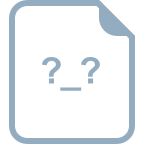
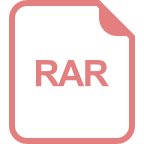
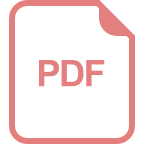
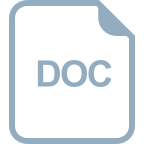













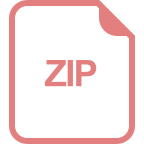