基于DEM数据 计算挖方量的C代码或C++代码
时间: 2023-11-28 22:05:04 浏览: 33
以下是基于DEM数据计算挖方量的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <cmath>
using namespace std;
// 读取DEM数据
void readDEMData(double** demData, const string& demFileName, const int& numRows, const int& numCols) {
ifstream inFile(demFileName.c_str(), ios::in | ios::binary);
if (!inFile) {
cout << "无法打开DEM文件!" << endl;
exit(1);
}
for (int i = 0; i < numRows; i++) {
for (int j = 0; j < numCols; j++) {
inFile.read((char*)&demData[i][j], sizeof(double));
}
}
inFile.close();
}
// 计算挖方量
double calculateExcavationVolume(double** demData, const int& numRows, const int& numCols, const double& cellSize, const double& baseHeight) {
double excavationVolume = 0.0;
for (int i = 0; i < numRows - 1; i++) {
for (int j = 0; j < numCols - 1; j++) {
double height1 = demData[i][j] - baseHeight;
double height2 = demData[i+1][j] - baseHeight;
double height3 = demData[i+1][j+1] - baseHeight;
double height4 = demData[i][j+1] - baseHeight;
double avgHeight = (height1 + height2 + height3 + height4) / 4.0;
double cellVolume = cellSize * cellSize * avgHeight;
excavationVolume += cellVolume;
}
}
return excavationVolume;
}
int main() {
string demFileName = "dem.bin";
int numRows = 1000;
int numCols = 1000;
double cellSize = 1.0;
double baseHeight = 0.0;
// 分配DEM数据空间
double** demData = new double*[numRows];
for (int i = 0; i < numRows; i++) {
demData[i] = new double[numCols];
}
// 读取DEM数据
readDEMData(demData, demFileName, numRows, numCols);
// 计算挖方量
double excavationVolume = calculateExcavationVolume(demData, numRows, numCols, cellSize, baseHeight);
cout << "挖方量为:" << excavationVolume << " 立方米" << endl;
// 释放DEM数据空间
for (int i = 0; i < numRows; i++) {
delete[] demData[i];
}
delete[] demData;
return 0;
}
```
其中,`dem.bin` 文件是以二进制格式存储的 DEM 数据文件,`numRows` 和 `numCols` 分别是 DEM 数据的行列数,`cellSize` 是 DEM 数据的单元大小,`baseHeight` 是计算基准高度(一般为原地面高程)。`readDEMData` 函数用于读取 DEM 数据,`calculateExcavationVolume` 函数用于计算挖方量。最后输出计算结果。注意,此代码仅供参考,实际使用中需根据实际情况进行修改。
相关推荐
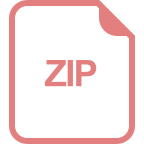
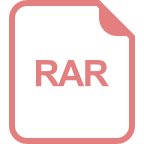














