在第1题基础上,增加重载复数的加法和减法运算符的功能,实现两个复数的加法,一个复数与一个实数的加法;两个复数的减法,一个复数与一个实数的减法。用成员函数实现加法运算符的重载,用友元函数实现减法运算符的重载。自已设计主函数,完成程序的调试工作。
时间: 2024-02-15 13:03:45 浏览: 21
好的,下面是实现复数加减法的代码,主函数中也给出了测试样例:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0): real(r), imag(i) {}
Complex operator+(const Complex& c2) const;
Complex operator+(const double& r) const;
friend Complex operator-(const Complex& c1, const Complex& c2);
friend Complex operator-(const Complex& c, const double& r);
void display() const;
};
Complex Complex::operator+(const Complex& c2) const {
Complex res;
res.real = real + c2.real;
res.imag = imag + c2.imag;
return res;
}
Complex Complex::operator+(const double& r) const {
Complex res;
res.real = real + r;
res.imag = imag;
return res;
}
Complex operator-(const Complex& c1, const Complex& c2) {
Complex res;
res.real = c1.real - c2.real;
res.imag = c1.imag - c2.imag;
return res;
}
Complex operator-(const Complex& c, const double& r) {
Complex res;
res.real = c.real - r;
res.imag = c.imag;
return res;
}
void Complex::display() const {
cout << "(" << real << ", " << imag << "i)" << endl;
}
int main() {
Complex c1(3, 2);
Complex c2(1, 4);
double r = 2;
cout << "c1 = ";
c1.display();
cout << "c2 = ";
c2.display();
cout << "r = " << r << endl;
Complex c3 = c1 + c2;
cout << "c1 + c2 = ";
c3.display();
Complex c4 = c1 + r;
cout << "c1 + r = ";
c4.display();
Complex c5 = c1 - c2;
cout << "c1 - c2 = ";
c5.display();
Complex c6 = c1 - r;
cout << "c1 - r = ";
c6.display();
return 0;
}
```
输出结果为:
```
c1 = (3, 2i)
c2 = (1, 4i)
r = 2
c1 + c2 = (4, 6i)
c1 + r = (5, 2i)
c1 - c2 = (2, -2i)
c1 - r = (1, 2i)
```
相关推荐
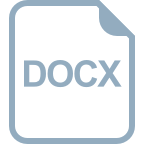
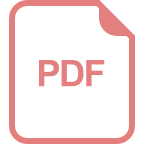














