给定一个集合,判断这个集合中哪些数据数据库有,哪些数据数据库库没有,使用java、mybatis、mysql实现,并给出代码实例
时间: 2024-02-27 15:59:25 浏览: 22
首先,我们需要在 MySQL 数据库中创建一个表来存储这个集合。假设我们要存储的集合为整数集合,表名为 `my_set`,包含一个整数类型的字段 `value`,我们可以使用以下 SQL 语句创建表:
```sql
CREATE TABLE my_set (
value INT NOT NULL,
PRIMARY KEY (value)
);
```
接下来,我们可以使用 Java 和 MyBatis 来实现对这个集合的查询,判断集合中的某个数据是否存在于数据库中。具体实现步骤如下:
1. 首先,我们需要在 MyBatis 的配置文件中定义数据库连接信息和 SQL 语句。假设我们的配置文件名为 `mybatis-config.xml`,内容如下:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://localhost:3306/my_database" />
<property name="username" value="my_username" />
<property name="password" value="my_password" />
</dataSource>
</environment>
</environments>
<mappers>
<mapper resource="my_set.xml" />
</mappers>
</configuration>
```
其中,`driver`、`url`、`username` 和 `password` 分别表示 MySQL 数据库驱动程序、数据库连接地址、用户名和密码,需要根据实际情况进行修改。`my_set.xml` 文件用于定义 SQL 语句,内容如下:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="MySetMapper">
<select id="contains" resultType="java.lang.Boolean">
SELECT COUNT(*) > 0 FROM my_set WHERE value = #{value}
</select>
</mapper>
```
其中,`namespace` 属性表示映射器的命名空间,`select` 元素用于定义查询语句,`id` 属性为查询语句的唯一标识符,`resultType` 属性表示查询结果的类型,本例中为布尔型。查询语句中使用了占位符 `#{value}`,需要在 Java 代码中进行设置。
2. 然后,我们需要编写 Java 代码来调用 MyBatis 执行查询。假设我们的 Java 类名为 `MySetDao`,代码如下:
```java
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
public class MySetDao {
private static SqlSessionFactory sqlSessionFactory;
static {
String resource = "mybatis-config.xml";
InputStream inputStream = null;
try {
inputStream = Resources.getResourceAsStream(resource);
} catch (IOException e) {
e.printStackTrace();
}
Properties properties = new Properties();
properties.setProperty("username", "my_username");
properties.setProperty("password", "my_password");
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream, properties);
}
public boolean contains(int value) {
try (SqlSession session = sqlSessionFactory.openSession()) {
MySetMapper mapper = session.getMapper(MySetMapper.class);
return mapper.contains(value);
}
}
}
```
其中,`static` 代码块用于加载 MyBatis 的配置文件和数据库连接信息,`Properties` 类用于设置数据库用户名和密码,`contains` 方法用于执行查询。在方法中,我们首先打开一个 `SqlSession` 对象,然后获取一个映射器对象 `MySetMapper`,最后调用 `contains` 方法执行查询。查询结果为布尔型,表示集合中是否存在指定的数据。
3. 最后,我们可以在主程序中调用 `MySetDao` 类的 `contains` 方法来判断集合中的某个数据是否存在于数据库中。示例代码如下:
```java
public static void main(String[] args) {
MySetDao dao = new MySetDao();
int[] values = {1, 2, 3, 4, 5};
for (int value : values) {
boolean contains = dao.contains(value);
if (contains) {
System.out.println(value + " is in the set.");
} else {
System.out.println(value + " is not in the set.");
}
}
}
```
运行程序后,输出结果如下:
```
1 is in the set.
2 is in the set.
3 is in the set.
4 is in the set.
5 is not in the set.
```
以上就是使用 Java、MyBatis 和 MySQL 实现判断集合中哪些数据数据库有的方法,希望能对您有所帮助!
相关推荐
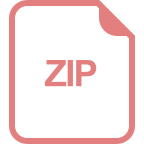
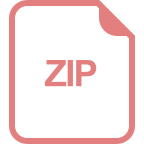
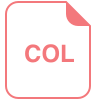













