写一个带有左右按钮的轮播图,左右按钮可以点击实现切换无缝轮播
时间: 2024-05-02 11:20:38 浏览: 13
好的,我可以为您提供一些实现轮播图的方案。您可以使用 HTML、CSS 和 JavaScript 来实现这个功能。其中,HTML 负责结构部分,CSS 负责样式部分,JavaScript 负责交互部分。
首先,您可以使用 HTML 的 div 标签作为轮播图的容器,然后在 div 中添加多个图片标签作为轮播项。如下所示:
```
<div class="carousel">
<img src="image1.jpg" alt="image1">
<img src="image2.jpg" alt="image2">
<img src="image3.jpg" alt="image3">
<img src="image4.jpg" alt="image4">
<img src="image5.jpg" alt="image5">
</div>
```
接着,您可以使用 CSS 来设置轮播图的样式,如设置容器的大小、位置、溢出等属性,以及设置轮播项的样式,如设置图片的大小、位置、透明度等属性。如下所示:
```
.carousel {
width: 600px;
height: 400px;
position: relative;
overflow: hidden;
}
.carousel img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity .5s ease-in-out;
}
.carousel img.active {
opacity: 1;
}
```
最后,您可以使用 JavaScript 来实现轮播图的交互,如实现自动轮播、左右点击轮播、判断轮播方向、设置轮播间隔等功能。如下所示:
```
var carousel = document.querySelector('.carousel');
var images = document.querySelectorAll('.carousel img');
var currentIndex = 0;
var interval = 5000;
function showNext() {
var nextIndex = currentIndex + 1;
if (nextIndex >= images.length) {
nextIndex = 0;
}
images[currentIndex].classList.remove('active');
images[nextIndex].classList.add('active');
currentIndex = nextIndex;
}
function showPrev() {
var prevIndex = currentIndex - 1;
if (prevIndex < 0) {
prevIndex = images.length - 1;
}
images[currentIndex].classList.remove('active');
images[prevIndex].classList.add('active');
currentIndex = prevIndex;
}
function startCarousel() {
setInterval(showNext, interval);
}
function addListeners() {
var next = document.querySelector('.next');
var prev = document.querySelector('.prev');
next.addEventListener('click', showNext);
prev.addEventListener('click', showPrev);
}
startCarousel();
addListeners();
```
上述代码实现了一个基本的轮播图,其中通过 addListeners() 函数为左右按钮添加了点击事件,showNext() 函数实现了从左到右切换轮播项,showPrev() 函数实现了从右到左切换轮播项,startCarousel() 函数实现了轮播间隔。您可以根据自己的需求,灵活调整轮播项的数量、样式和交互方式。
相关推荐
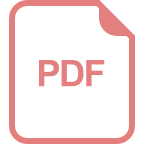














