原生js轮播图 要求左右按钮 小圆点点击
时间: 2023-09-05 09:09:52 浏览: 175
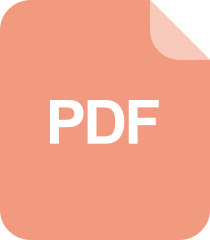
原生JS实现图片左右轮播
以下是一个简单的原生 JavaScript 轮播图实现,包含左右按钮和小圆点点击功能:
HTML 代码:
```html
<div class="slider">
<div class="slider-wrapper">
<img src="https://via.placeholder.com/600x400?text=Slide%201" alt="Slide 1">
<img src="https://via.placeholder.com/600x400?text=Slide%202" alt="Slide 2">
<img src="https://via.placeholder.com/600x400?text=Slide%203" alt="Slide 3">
</div>
<div class="slider-controls">
<button class="slider-control slider-control-prev"><</button>
<button class="slider-control slider-control-next">></button>
<div class="slider-dots">
<button class="slider-dot"></button>
<button class="slider-dot"></button>
<button class="slider-dot"></button>
</div>
</div>
</div>
```
CSS 代码:
```css
.slider {
position: relative;
width: 600px;
height: 400px;
margin: 0 auto;
overflow: hidden;
}
.slider-wrapper {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
display: flex;
transition: transform 0.5s ease-in-out;
}
.slider-wrapper img {
width: 100%;
height: 100%;
object-fit: cover;
}
.slider-controls {
position: absolute;
bottom: 0;
left: 0;
width: 100%;
display: flex;
justify-content: center;
align-items: center;
}
.slider-control {
font-size: 2rem;
font-weight: bold;
color: white;
background-color: rgba(0, 0, 0, 0.3);
border: none;
cursor: pointer;
margin: 0 0.5rem;
padding: 0.5rem;
border-radius: 50%;
}
.slider-dot {
width: 1rem;
height: 1rem;
background-color: rgba(255, 255, 255, 0.5);
border: none;
cursor: pointer;
margin: 0 0.5rem;
padding: 0;
border-radius: 50%;
}
.slider-dot.active {
background-color: white;
}
```
JavaScript 代码:
```js
const slider = document.querySelector('.slider');
const sliderWrapper = document.querySelector('.slider-wrapper');
const slides = Array.from(sliderWrapper.children);
const prevButton = document.querySelector('.slider-control-prev');
const nextButton = document.querySelector('.slider-control-next');
const dots = Array.from(document.querySelectorAll('.slider-dot'));
let slideWidth = slider.clientWidth;
let currentSlide = 0;
// Set initial position of slides
slides.forEach((slide, index) => {
slide.style.left = index * slideWidth + 'px';
});
// Move to the next slide
function moveToNextSlide() {
if (currentSlide === slides.length - 1) {
currentSlide = 0;
} else {
currentSlide++;
}
moveSlides();
}
// Move to the previous slide
function moveToPrevSlide() {
if (currentSlide === 0) {
currentSlide = slides.length - 1;
} else {
currentSlide--;
}
moveSlides();
}
// Move to a specific slide
function moveToSlide(index) {
currentSlide = index;
moveSlides();
}
// Move the slides to the current slide
function moveSlides() {
sliderWrapper.style.transform = `translateX(-${currentSlide * slideWidth}px)`;
updateDots();
}
// Update the active dot
function updateDots() {
dots.forEach((dot, index) => {
if (index === currentSlide) {
dot.classList.add('active');
} else {
dot.classList.remove('active');
}
});
}
// Handle next button click
nextButton.addEventListener('click', moveToNextSlide);
// Handle previous button click
prevButton.addEventListener('click', moveToPrevSlide);
// Handle dot button click
dots.forEach((dot, index) => {
dot.addEventListener('click', () => {
moveToSlide(index);
});
});
// Handle window resize
window.addEventListener('resize', () => {
slideWidth = slider.clientWidth;
moveSlides();
});
// Move to the first slide
moveToSlide(0);
```
说明:
- 首先获取轮播图容器和相关元素。
- 设置初始位置和宽度。
- 实现移动到下一个、前一个和指定的幻灯片的函数。
- 实现移动幻灯片和更新圆点的函数。
- 添加按钮和圆点的事件监听器。
- 处理窗口大小调整事件。
- 移动到第一张幻灯片。
注意:此代码仅用于教学目的,实际使用可能需要进行更多的优化和改进。
阅读全文
相关推荐
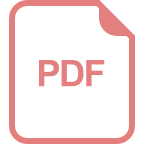
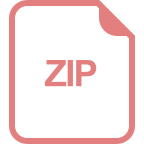
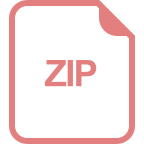
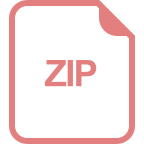
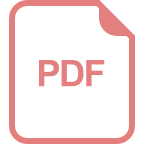
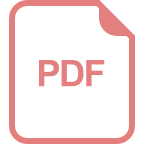
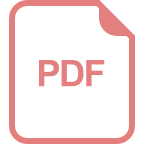
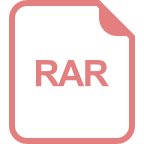
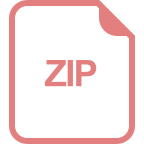
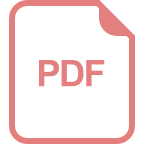
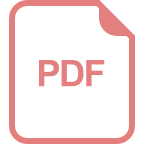
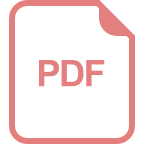
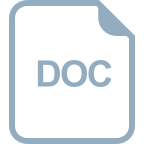
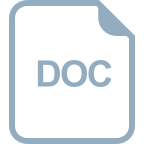
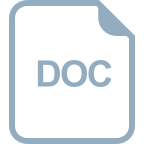
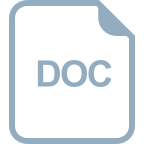
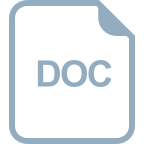