用c++,visual studio,写一段能够运行的程序,使它能够模拟点单系统
时间: 2024-03-19 13:42:01 浏览: 38
好的,我可以帮你写一个简单的点单系统程序,使用 C++ 编写,可以在 Visual Studio 中运行。
首先,我们需要定义一些数据类型和变量来存储菜单项和订单信息:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 菜单项
struct MenuItem {
string name; // 名称
double price; // 价格
};
// 订单项
struct OrderItem {
string name; // 名称
int quantity; // 数量
};
// 所有菜单项
vector<MenuItem> menu = {
{"汉堡", 15.0},
{"薯条", 8.0},
{"可乐", 5.0}
};
// 当前订单
vector<OrderItem> order;
```
然后,我们可以定义一些函数来处理用户输入和输出订单信息:
```c++
// 显示菜单
void showMenu() {
cout << "菜单:" << endl;
for (int i = 0; i < menu.size(); i++) {
cout << i + 1 << ". " << menu[i].name << " ¥" << menu[i].price << endl;
}
}
// 显示订单
void showOrder() {
double total = 0.0;
cout << "订单:" << endl;
for (int i = 0; i < order.size(); i++) {
double price = 0.0;
for (int j = 0; j < menu.size(); j++) {
if (menu[j].name == order[i].name) {
price = menu[j].price;
break;
}
}
cout << order[i].name << " x " << order[i].quantity << " ¥" << price * order[i].quantity << endl;
total += price * order[i].quantity;
}
cout << "总价:¥" << total << endl;
}
// 处理用户输入
void handleInput() {
int choice;
cout << "请输入菜单编号,或者输入 0 结束点单:" << endl;
cin >> choice;
while (choice != 0) {
if (choice < 1 || choice > menu.size()) {
cout << "无效的菜单编号,请重新输入:" << endl;
} else {
string name = menu[choice - 1].name;
int quantity;
cout << "请输入数量:" << endl;
cin >> quantity;
if (quantity < 1) {
cout << "数量不能为零,请重新输入:" << endl;
} else {
bool found = false;
for (int i = 0; i < order.size(); i++) {
if (order[i].name == name) {
order[i].quantity += quantity;
found = true;
break;
}
}
if (!found) {
order.push_back({name, quantity});
}
showOrder();
}
}
cout << "请输入菜单编号,或者输入 0 结束点单:" << endl;
cin >> choice;
}
}
```
最后,我们可以编写主函数来调用上述函数:
```c++
int main() {
showMenu();
handleInput();
return 0;
}
```
这样,我们就完成了一个简单的点单系统程序。用户可以通过输入菜单编号和数量来点单,并且可以随时查看订单和总价。
阅读全文
相关推荐
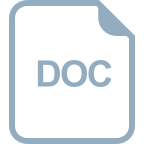
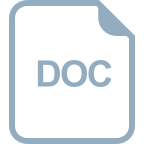
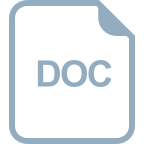
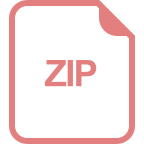
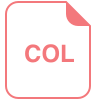
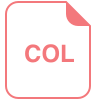
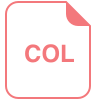
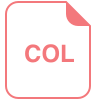
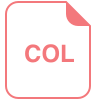
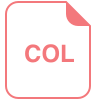
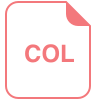
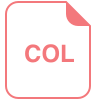
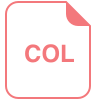
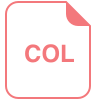
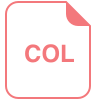
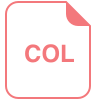
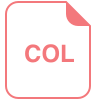
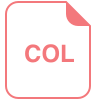