vue 高德地图 label API
时间: 2023-10-12 19:14:56 浏览: 165
Vue 高德地图中的 Label API 可以用来在地图上添加标注,标注上可以显示文本信息。下面是一个简单的示例:
1. 首先在 Vue 组件中引入高德地图的 JS API:
```
<script src="https://webapi.amap.com/maps?v=1.4.15&key=您申请的key值"></script>
```
2. 在 Vue 组件的 mounted 钩子函数中初始化地图和 Label:
```
mounted() {
// 初始化地图
const map = new AMap.Map('map-container', {
zoom: 14,
center: [116.39, 39.9]
})
// 添加 Label
const label = new AMap.Marker({
position: [116.39, 39.9],
map: map,
label: {
content: '这里是标注的文本内容',
offset: new AMap.Pixel(20, 20)
}
})
}
```
在上面的代码中,我们首先初始化了一个地图实例,并设置了地图的缩放级别和中心点坐标。然后,在地图上添加了一个 Label,设置了标注的位置、地图实例、文本内容和偏移量。
需要注意的是,Label API 还有很多其他的配置项可以使用,比如字体颜色、字体大小等,更多的信息可以参考高德地图的官方文档。
相关问题
echarts迁徙图 vue_vue中高德搭配echarts做数据迁徙流线图
实现 Vue 中高德地图搭配 Echarts 迁徙图的步骤如下:
1. 安装依赖
```
npm install echarts vue-echarts vue-amap --save
```
2. 在 main.js 中引入依赖
```javascript
import Vue from 'vue'
import VueAMap from 'vue-amap'
import ECharts from 'vue-echarts'
// 引入 ECharts 主题和扩展模块
import 'echarts/lib/chart/map'
import 'echarts/lib/chart/effectScatter'
import 'echarts/lib/component/tooltip'
import 'echarts/lib/component/title'
import 'echarts/lib/component/legend'
// 注册 ECharts 组件
Vue.component('v-chart', ECharts)
// 初始化高德地图插件
Vue.use(VueAMap)
VueAMap.initAMapApiLoader({
key: '你的高德地图 api key',
plugin: ['AMap.DragRoute']
})
```
3. 在组件中使用
```vue
<template>
<div>
<v-chart class="chart" :options="options" />
</div>
</template>
<script>
export default {
data() {
return {
options: {
tooltip: {
trigger: 'item'
},
legend: {
orient: 'vertical',
top: 'bottom',
left: 'right',
data: ['流入', '流出']
},
series: [
{
name: '迁徙',
type: 'lines',
zlevel: 1,
effect: {
show: true,
period: 6,
trailLength: 0.7,
color: '#fff',
symbolSize: 3
},
lineStyle: {
normal: {
color: '#a6c84c',
width: 0,
curveness: 0.2
}
},
data: [
{
fromName: '北京',
toName: '上海',
coords: [
[116.407394, 39.904211],
[121.473662, 31.230372]
]
},
{
fromName: '北京',
toName: '广州',
coords: [
[116.407394, 39.904211],
[113.280637, 23.125178]
]
}
]
},
{
name: '流入',
type: 'map',
mapType: 'china',
roam: false,
label: {
normal: {
show: true
},
emphasis: {
show: true
}
},
itemStyle: {
normal: {
borderWidth: 0.5,
borderColor: '#009fe8',
areaColor: '#fff'
},
emphasis: {
areaColor: '#009fe8'
}
},
data: [
{ name: '北京', value: 100 },
{ name: '上海', value: 200 },
{ name: '广州', value: 300 }
]
},
{
name: '流出',
type: 'map',
mapType: 'china',
roam: false,
label: {
normal: {
show: true
},
emphasis: {
show: true
}
},
itemStyle: {
normal: {
borderWidth: 0.5,
borderColor: '#009fe8',
areaColor: '#fff'
},
emphasis: {
areaColor: '#009fe8'
}
},
data: [
{ name: '北京', value: 50 },
{ name: '上海', value: 100 },
{ name: '广州', value: 150 }
]
}
]
}
}
}
}
</script>
```
以上代码实现了一个简单的迁徙图,其中 `fromName` 和 `toName` 表示迁徙的起点和终点,`coords` 表示起点和终点的经纬度。`flowIn` 和 `flowOut` 表示流入和流出的数据,可以根据实际需求进行修改。
Vue2中使用new L.GeoMap构建地图怎么添加地地图搜索功能
在Vue 2中,要使用`L.GeoMap`构建地图并添加地图搜索功能,通常你需要结合`Leaflet.markercluster`库来创建标记群组,并使用`Leaflet.MarkerWithLabel`或类似插件显示搜索结果的标签。以下是一个简单的步骤:
1. 首先,确保已经安装了相关的依赖:
```
npm install leaflet leaflet.markercluster leaflet.markerwithlabel vue-leaflet
```
2. 在你的Vue组件中引入这些库:
```javascript
import L from 'leaflet';
import MarkerCluster from 'leaflet.markercluster';
import MarkerWithLabel from 'leaflet.markerwithlabel';
```
3. 初始化地图和地图容器:
```html
<div id="map" ref="map"></div>
```
4. 在`data()`方法中配置地图:
```javascript
data() {
return {
map: null,
markers: []
};
},
mounted() {
this.initMap();
},
methods: {
initMap() {
const bounds = L.latLngBounds(
// 设置地图中心点和初始缩放级别
[51.505, -0.09], // 北京坐标示例
[51.705, -0.19]
);
this.map = L.map('map').setView(bounds.getCenter(), bounds.getZoom());
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {}).addTo(this.map);
}
}
```
5. 添加地图搜索功能可以借助第三方服务如Google Places API、高德地图API等。这里仅给出一个通用思路,实际操作时需要替换为你选择的服务的API和回调:
```javascript
methods: {
searchLocation(query) {
fetch(`your_search_api_url?q=${query}`)
.then(response => response.json())
.then(data => {
// 对数据进行处理,提取经纬度信息
const locations = data.map(result => ({
lat: result.geometry.location.lat(),
lng: result.geometry.location.lng()
}));
// 创建marker实例并添加到地图上
locations.forEach(location => {
const marker = new MarkerWithLabel({
position: [location.lat, location.lng],
draggable: false,
icon: L.divIcon({ className: 'marker-label-icon' }),
labelContent: query,
riseOnHover: true
});
this.markers.push(marker);
marker.addTo(this.map);
// 如果使用MarkerCluster,你可以将单个marker转换为ClusterGroup
// const clusterGroup = new MarkerCluster().addLayers([marker]);
// clusterGroup.addTo(this.map);
});
})
.catch(error => console.error(error));
}
}
```
6. 用户触发搜索事件(例如输入框改变或按钮点击),调用`searchLocation`方法:
```html
<input v-model="query" @input="searchLocation($event.target.value)">
```
记得在`mounted`钩子之外的地方获取地图,因为此时地图已经初始化完毕。
阅读全文
相关推荐
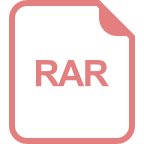
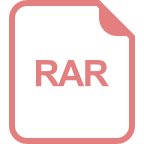
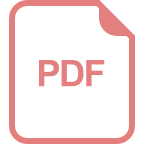



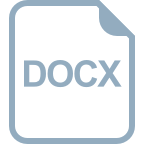
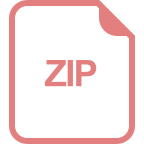