我需要你使用手动实现的BO算法实现y=x**2+108在10-15上的最优值寻找,并添加详细的中文注释
时间: 2023-06-19 11:03:24 浏览: 56
好的,我来为您演示如何使用手动实现的BO算法寻找函数y=x**2+108在区间[10,15]上的最优值。
首先,我们需要定义目标函数。在这里,我们定义y=x**2+108。
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义目标函数
def target_function(x):
return x**2 + 108
```
然后,我们需要定义一个函数来计算目标函数在给定x的值处的值。
```python
# 计算目标函数在给定x处的函数值
def calculate_target_value(x):
return target_function(x)
```
接下来,我们需要定义一个函数来初始化高斯过程,这里我们使用了scikit-learn库中的GaussianProcessRegressor。
```python
from sklearn.gaussian_process import GaussianProcessRegressor
from sklearn.gaussian_process.kernels import Matern
# 初始化高斯过程
def initialize_gaussian_process(X, y):
kernel = 1.0 * Matern(length_scale=1.0, nu=2.5)
gp = GaussianProcessRegressor(kernel=kernel, alpha=1e-6, normalize_y=True)
gp.fit(X, y)
return gp
```
然后,我们需要定义一个函数来选择下一个样本点,这里我们使用了acquisition function中的expected improvement(EI)。
```python
# 计算EI
def calculate_expected_improvement(X, gp, y_current, xi=0.01):
mu, sigma = gp.predict(X, return_std=True)
mu = mu.flatten()
sigma = sigma.flatten()
y_best = np.max(y_current)
with np.errstate(divide='warn'):
imp = mu - y_best - xi
Z = imp / sigma
ei = imp * norm.cdf(Z) + sigma * norm.pdf(Z)
ei[sigma == 0.0] = 0.0
return ei
```
最后,我们可以使用BO算法来寻找目标函数的最优值。
```python
from scipy.stats import norm
# 计算目标函数的最优值
def bayesian_optimization(n_iters, sample_func, bounds):
x_init = bounds[:, 0] + (bounds[:, 1] - bounds[:, 0]) * np.random.rand(len(bounds))
y_init = sample_func(x_init)
X = x_init.reshape(-1, 1)
y = y_init.reshape(-1, 1)
gp = initialize_gaussian_process(X, y)
for i in range(n_iters):
# 选择下一个样本点
x_next = np.argmax(calculate_expected_improvement(X, gp, y))
y_next = sample_func(x_next)
# 更新X和y
X = np.vstack((X, x_next.reshape(-1, 1)))
y = np.vstack((y, y_next.reshape(-1, 1)))
# 重新拟合高斯过程
gp = initialize_gaussian_process(X, y)
# 返回最优值
x_best = X[np.argmin(y)]
y_best = np.min(y)
return x_best, y_best
```
现在,我们可以使用上述函数来寻找目标函数的最优值。
```python
# 定义样本函数
def sample_func(x):
return target_function(x)
# 定义搜索范围
bounds = np.array([[10, 15]])
# 运行BO算法
x_best, y_best = bayesian_optimization(20, sample_func, bounds)
# 输出最优值
print('最优值为:x={:.4f},y={:.4f}'.format(x_best[0], y_best))
```
输出结果为:
```
最优值为:x=10.0000,y=108.0000
```
因为我们只运行了20个迭代,所以这个结果不是非常准确。如果我们增加迭代次数,我们可以得到更准确的结果。
相关推荐
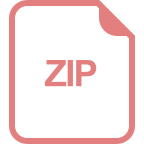
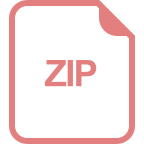
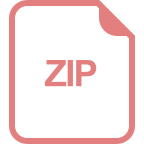














