3、随机产生300个整数构成的序列,分别以直接插入、冒泡、希尔、快速、归并等排序算法排序,并统计各自的比较次数。
时间: 2023-10-20 20:14:14 浏览: 79
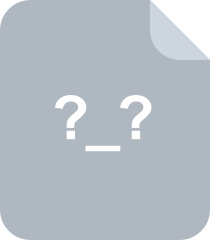
插入排序 冒泡法排序 快速排序 直接选择排序 堆排序 归并排序 希尔排序 7种排序算法及时间比较

好的,我来为你编写这个程序。首先,我们需要先生成一个包含300个随机整数的序列:
```python
import random
lst = [random.randint(1, 1000) for _ in range(300)]
```
接下来,我们可以定义直接插入排序算法:
```python
def insertion_sort(lst):
count = 0
for i in range(1, len(lst)):
j = i
while j > 0 and lst[j] < lst[j-1]:
lst[j], lst[j-1] = lst[j-1], lst[j]
j -= 1
count += 1
return count
```
然后定义冒泡排序算法:
```python
def bubble_sort(lst):
count = 0
for i in range(len(lst)-1):
for j in range(len(lst)-1-i):
if lst[j] > lst[j+1]:
lst[j], lst[j+1] = lst[j+1], lst[j]
count += 1
return count
```
接下来是希尔排序算法:
```python
def shell_sort(lst):
count = 0
gap = len(lst) // 2
while gap > 0:
for i in range(gap, len(lst)):
temp = lst[i]
j = i
while j >= gap and lst[j-gap] > temp:
lst[j] = lst[j-gap]
j -= gap
count += 1
lst[j] = temp
gap //= 2
return count
```
然后是快速排序算法:
```python
def quick_sort(lst):
count = 0
if len(lst) <= 1:
return lst, count
pivot = lst[0]
left = []
right = []
for i in range(1, len(lst)):
if lst[i] < pivot:
left.append(lst[i])
count += 1
else:
right.append(lst[i])
count += 1
sorted_left, count_left = quick_sort(left)
sorted_right, count_right = quick_sort(right)
count += count_left + count_right
return sorted_left + [pivot] + sorted_right, count
```
最后是归并排序算法:
```python
def merge_sort(lst):
count = 0
if len(lst) <= 1:
return lst, count
mid = len(lst) // 2
left = lst[:mid]
right = lst[mid:]
sorted_left, count_left = merge_sort(left)
sorted_right, count_right = merge_sort(right)
count += count_left + count_right
i = j = 0
merged = []
while i < len(sorted_left) and j < len(sorted_right):
if sorted_left[i] < sorted_right[j]:
merged.append(sorted_left[i])
i += 1
else:
merged.append(sorted_right[j])
j += 1
count += 1
merged += sorted_left[i:]
merged += sorted_right[j:]
return merged, count
```
最后,我们可以运行这些排序算法,并打印出每个算法的比较次数:
```python
lst_copy = lst.copy()
count = insertion_sort(lst_copy)
print("直接插入排序比较次数:", count)
lst_copy = lst.copy()
count = bubble_sort(lst_copy)
print("冒泡排序比较次数:", count)
lst_copy = lst.copy()
count = shell_sort(lst_copy)
print("希尔排序比较次数:", count)
lst_copy = lst.copy()
sorted_lst, count = quick_sort(lst_copy)
print("快速排序比较次数:", count)
lst_copy = lst.copy()
sorted_lst, count = merge_sort(lst_copy)
print("归并排序比较次数:", count)
```
这就是完整的程序了,你可以在本地运行它并查看结果。
阅读全文
相关推荐
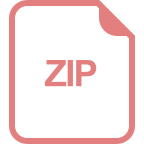








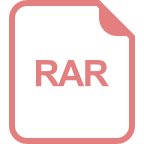
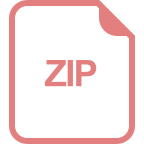
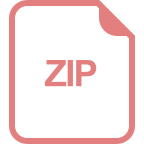
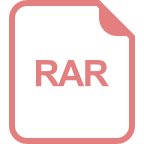
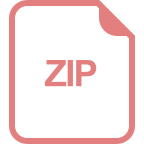